Section 3.6 Graphic Shape Objects
Subsection 3.6.1 Shapes and Other Classes
The DoodlePad library provides several classes that you can instantiate just like String, Scanner, and Random. Shape classes include:
Class | Description |
---|---|
Oval |
An oval or ellipse shape |
Rectangle |
A rectangle shape |
Line |
A straight line shape |
Arc |
A curved segment from an ellipse edge |
Text |
A shape that displays a String as a graphic |
RoundRect |
A rectangle shape with rounded corners |
Polygon |
A polygon with arbitrary straight-line edges |
Path |
A more complex shape made of edges that are both straight and curved |
Image |
A shape that displays an image and/or may be programmatically drawn upon |
Sprite |
A shape that sequentially shows frames of a short animation |
Other helper classes included in the library include:
Class | Description |
---|---|
Timer |
Periodically invoke another method, like advancing Sprite frames |
Sound |
Loads and plays a sound file |
Each class has its own constructors and a selection of other methods that you can use to create Shapes and work with them.
The classes in the DoodlePad library are not available to your Java program by default. They are included in a library JAR file (Java ARchive) named
doodlepad.jar
, which you may download directly from the GitHub repository 1 .It is most convenient to copy the
doodlepad.jar
file into the same folder as your Java source code file.Like other classes in Java, to access the Shape classes in the file, you must import the classes. You can import all DoodlePad classes by adding the following line to the top of your Java program. We’ll demonstrate this in the next section.
import doodlepad.*;
Subsection 3.6.2 Creating Shape Objects
Shape constructors can vary, so it is always a good idea to look up the available constructors provided by a specific shape class by referring to the library documentation 2 . Nevertheless, many Shape class constructors have signatures that take four parameter values:
x
: x-coordinate if Shape’s upper-left corner(double)
y
: y-coordinate if Shape’s upper-left corner(double)
width
: Shape width(double)
height
: Shape height(double)
The Text class constructor takes three parameter values, the String to display and the (x, y) location on the Window where to draw the Text. For example, the following small program instantiates several Shape objects, which are displayed automatically. Note how the following constructor inocations follow the standard format.
// Shapes.java
import doodlepad.*;
public class Shapes
{
public static void main(String[] args)
{
Oval o = new Oval(100, 10, 50, 40); // Create an Oval object
Rectangle r = new Rectangle(100, 70, 60, 30); // Create a Rectangle object
Text t = new Text("Hello World", 100, 120);// Create a Text object
}
}
Shapes.java
To compile this program, first make sure that both the
Shapes.java
and doodlepad.jar
file are in your folder. Open a terminal and make sure it is set to the same folder that contains your files. (Refer to the cd
shell command in Appendix B.)The command to compile your program is the same on all operating systems and shells. Use the following command to compile your program file to produce the
Shapes.class
file. The -cp
options is short for --class-path
, and is used to tell the command where to look for additional Java classes. Because DoodlePad Shape classes are not part of the Java Core Library, this command must be informed where to find the additional classes.javac -cp doodlepad.jar Shapes.java
The command to launch your compiled program vary slightly from platform to platform. These are listed in Table 3.6.4. Refer to Section 14.1 for a lot more detail on how to interpret these commands.
Operating System | Shell | Launch Command |
---|---|---|
Windows | Command Prompt | java -cp .;doodlepad.jar Shapes |
Windows | Powershell | java -cp .`;doodlepad.jar Shapes |
macOS & Linux | bash | java -cp .:doodlepad.jar Shapes |
After launching the compiled
Shapes.java
program you will see an output like that in Figure 3.6.5. Note that to display each shape you only needed to create the object instance. Everything else is handled for you, automatically.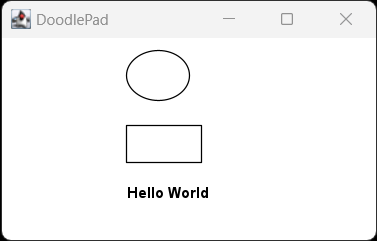
Shapes.java
Subsection 3.6.3 Styling Shape Objects
Shape objects share many methods that may be used for styling Shapes, such as setting fill color, stroke (outline) color, stroke width, etc. All color components in DoodlePad use the RGB (red-green-blue) or RGBA (red-green-blue-alpha) color models. Refer to Table 14.5.4 and the Listing 3.6.7 for examples. As with all objects, invoking methods involves following the object variable with a dot, the method to be invoked, and parentheses containing a comma-separated list of arguments to pass to the method’s parameters.
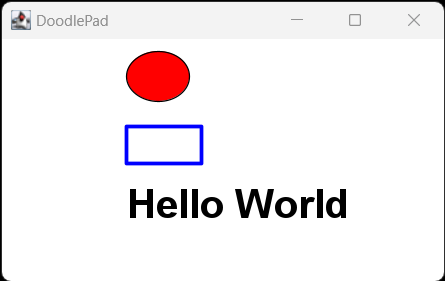
Styles.java
// Styles.java
import doodlepad.*;
public class Styles {
public static void main(String[] args) {
Oval o = new Oval(100, 10, 50, 40); // Create an Oval object
Rectangle r = new Rectangle(100, 70, 60, 30); // Create a Rectangle object
Text t = new Text("Hello World", 100, 120);// Create a Text object
o.setFillColor(255, 0, 0); // Red Oval
r.setStrokeWidth(3); // Thick blue outline
r.setStrokeColor(0, 0, 255); // for Rectangle
t.setFontSize(32); // Text font size
}
}
Styles.java
github.com/russomf/doodlepad/blob/master/dist/doodlepad.jar?raw=true
doodlepad.org/dist/javadoc/doodlepad/package-summary.html