Section 6.1 for
Statements
In Section 5.3 we learned that the three important steps for a while-statement configured for counting were:
- A counter variable is declared and initialized before the while-statement.
- The counter variable is incremented within the block of code associated with the while-statement.
- The while-statement’s conditional test checks if the counter exceeds some value and stops.
A while-statement configured for counting turns out to be such an important control structure that a special statement was introduced whose job is dedicated primarily to the execution of a block of code for a fixed number of iterations. This is the for-statement. The following diagram shows the parts of a for-statement.
Similar to the while- and if-statements, the for-statement starts with a special keyword (
for
), and is followed by a pair of parentheses containing expressions and a code block delimited by curly braces. An important difference between the for-statement and the while-statement is that the for-statement contains three expressions between its parentheses, not one. The three expressions are the initializer, test for continuation, and update, all separated with semicolons (;
). The columns of Table 6.1.2 describe each in more detail.Initializer | Continuation Test | Update | |
---|---|---|---|
Position | left | center | right |
Description | Declares and/or initializes a counter |
Checks if the next iteration should proceed |
Increments a counter or other expression |
Executes | Once at the start of an if-statement |
Before each iteration begins |
after each iteration ends |
Example | int i = 0 |
i < 10 |
i++ |
A for-statement is useful when iterating a fixed number of times, and is preferred over a while-statement whenever possible due to its compactness and clarity. Also, the
break
and continue
statements work with a for-statement in manner identical to the while-statement. (See Section 5.4).
Listing 6.1.3 is a rewrite of the example in Listing 5.6.5 with while-statements replaced by for-statements. Notice how the elements of the program responsible for iteration are more compact, and easier to read now that the three expressions are grouped together within the parentheses following the
for
keyword.// Grid4.java
import doodlepad.*; // Import graphics
public class Grid4 {
public static void main(String[] args) { // Start execution
Rectangle r; // Helper variables
boolean flip = false;
for (int x = 5; x <= 550; x += 120) { // x-coordinate loop
for (int y = 5; y <= 550; y += 60) {// y-coordinate loop
if (x == y) { continue; } // Skip when x == y
r = new Rectangle(x, y, 110, 50); // New Rectangle
flip = !flip; // flip-flop
if (flip) { // If flip
r.setFillColor(0, 0, 255); // Fill blue
} else { // If flop
r.setFillColor(255, 215, 0); // Fill gold
}
}
}
}
}
With the extra space available, we modified the logic a bit. Rather than instantiate new Rectangle when
x != y
, we are now performing the complementary action. When x == y
we invoke continue
which terminates the iteration and returns immediately back to the for-statement to check for another iteration. Note that it would have been tricky to use the same approach with while-statements. In the while-statement our loop counter was incremented at the end of the code block. If we executed a continue
before the end of the code block the loop counter would have never been updated. This would cause the program to enter an infinite loop, and never terminate. With a for-statement, the increment is always executed, whether or not the code block completes. This make the continue
statement much more convenient to use.Another update we made in
Grid4.java
is the addition of fill colors for the Rectangles. We wanted to fill every other Rectangle with blue or gold. To achieve this we declared a boolean
variable named flip
. Each time through the inner code block, we negate flip
with the statement flip = !flip
. In other words, if flip == true
then it becomes false
. If flip == false
then it becomes true
. We used this flip-flop variable and an if-statement to decide which color to use to fill the current Rectangle.javac -cp doodlepad.jar Grid4.java java -cp .;doodlepad.jar Grid4
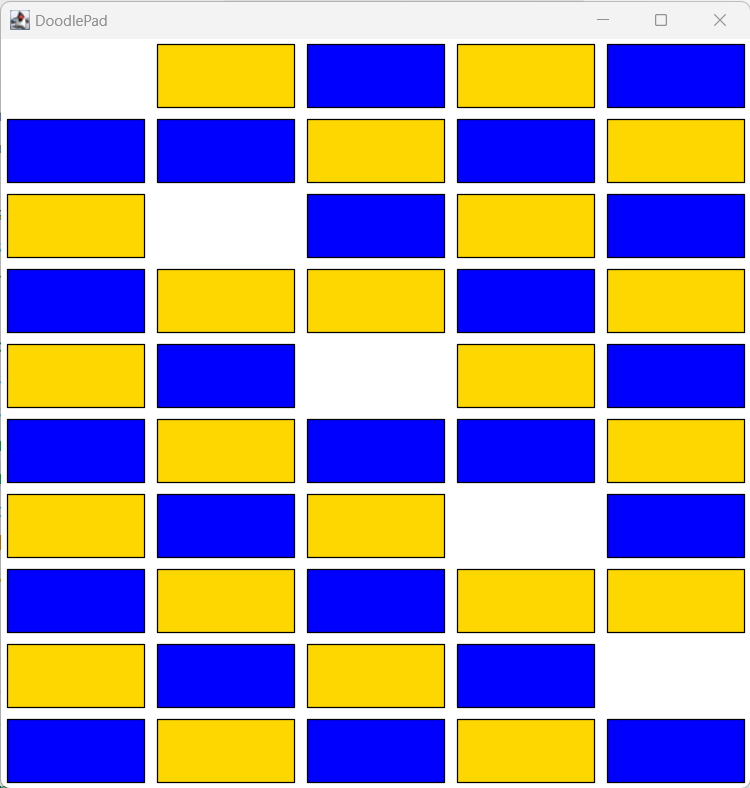
Grid4.java
There is one more item to note about for-statements associated with its block scope. In Section 5.7 we introduced the concept of block scope and mentioned how all statements having a code block define a new block scope. This is the case with for-statements as well. If we define a variable within the code block of a for-statement, that variable may not be accessed from outside the block scope.
With a for-statement, not only are declarations within the curly braces considered to exist within the scope of the code block, but any declarations within the parentheses also are considered to exist within the scope of the code block. To demonstrate, let’s revisit Listing 5.7.1 and update it with a for-statement having a loop counter
i
that is declared when initialized.// BlockScope2.java
import doodlepad.*;
public class BlockScope2 {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) { // Create 10 Ovals
Oval o = new Oval();
}
System.out.println( i ); // Print final value of i
}
}
When we attempt to compile this program, the compiler is unable to find the our loop counter variable
i
because it is declared within the parentheses of the for-statement, and therefore is considered to exist in that statement’s block scope. (See below.) If you do have a need to access i
after the for-statement, you can circumvent this problem by declaring i
before the for-statement, and use the first expression in the parentheses for initializing i
only, without the declaration.javac -cp doodlepad.jar BlockScope2.java BlockScope2.java:9: error: cannot find symbol System.out.println( i ); // Print final value of i ^ symbol: variable i location: class BlockScope2 1 error