Section 9.3 Method Overriding
A method invoked on a derived class that does not implement the exact method signature, will cause Java to search for the method signature in the base class, and on up the inheritance hierarchy if necessary. But, if a method with the signature is implemented in the dervied class, then that implementation will be the one that is invoked, even if the same method signature has been implemented in a base class. When a method in a dervied class has the same signature as one in its base class, we say that the derived class method overrides the one in the base class.
Subsection 9.3.1 Applications of Method Overriding
It may be the case that a derived class shares many of the behaviors of a base class, but not all. The methods that differ may be reimplemented correctly in the derived class. Because the search for a particular method signature moves up an inheritance hierarchy, overriding a method in a derived class effectively replaces it.
In another scenario, a base class method may constitute only part of what a derived class needs to be performed, but not all. In this case the derived class can override the base class method, and augment its behavior by invoking the base class method and adding to it. This could be as simple as logging the fact that the base class method signature is overridden by the derived class method by adding a print statement.
In a third application, a derived class might override a base class method as a way of stopping its execution, and simply returning. This might be used when a derived class is designed to simulate the behavior of a base class during testing. If certain base class methods are expensive to execute, we can use a derived class to catch and surpress the execution of certain base class methods during testing, and then remove the derived class before final release.
Whether replacing, augmenting, or surpressing base class methods, method overriding is a powerful way to modify base class behavior, forming a specialized derived class.
Subsection 9.3.2 Overriding Event Handler Methods
You are familiar with how DoodlePad’s graphic objects can handle events such as a mouse click or key press. In Subsection 14.6.1 we learned how to associate a method with an event by passing a method reference to a special method such as
setMousePressedHandler(…)
. DoodlePad provides a second way to handle events. When extending a shape or the Pad class, you may override certain inherited methods as a way to handle associated events rather than assigning a method reference. This approach can be more convenient and intuitive to implement.Let’s do a better job at implementing our ToggleButton class, which we first created in Listing 14.6.4. Now that we’re familiar with what we can do using inheritance, let’s reconsider ToggleButton’s implementation. Refer to Listing 9.3.1 for all the details of the updated class.
// ToggleButton.java
import doodlepad.*;
// ToggleButton class extends RoundRect
public class ToggleButton extends RoundRect {
private boolean on; // Button state
// Constructor
public ToggleButton(double x, double y, double w, double h) {
super(x, y, w, h, 20, 20);
this.on = false; // Starts off
this.setFillColor(200);
this.setText("Off");
}
// Handle button click event using inheritance
@Override
public void onMousePressed(double x, double y, int btn) {
super.onMousePressed(x, y, btn); // invoke default behavior
this.toggle(); // Toggle button
}
// Toggle button
public void toggle() {
this.on = !this.on; // Flip state
if (this.on) { // Update graphic
this.setFillColor(0, 255, 0);
this.setText("On");
} else {
this.setFillColor(200);
this.setText("Off");
}
}
// Test the class
public static void main(String[] args) {
// Create a new ToggleButton
ToggleButton myButton = new ToggleButton(100, 100, 75, 50);
}
}
ToggleButton.java
(version 2)This new implementation of
ToggleButton
takes advantage of inheritance by extending the RoundRect class instead of managing an internal RoundRect object. With this update we can now delete the RoundRect object instance variable used in Listing 14.6.4. The new constructor is modified by first removing the statement to initialize the shpButton
instance variable and replace it with a statement that invokes the base class constructor using super(…)
. The new constructor was expanded to take the button’s location and size. These parameter values are relayed to the base class constructor as parameters passed to super(…)
. Additionally, the former constructor is modified by removing the setMousePressedHandler(…)
method entirely, which is no longer needed because we now use method overriding to handle the mouse-pressed event.The former
onPressed(…)
event handler method is renamed and its parameters were changed to match the signature onMousePressed(double x, double y, int btn)
exactly. This is the signature of the base class method, and must be matched in order to override it with the derived class method. We add the Java compiler directive @Override
before the method as a precaution. This directive tells the compiler that we intend to override a base class method with the onMousePressed(…)
method declaration and to please check it. If the method does not override a base class method, then please throw a compiler error. Using the @Override
directive is highly recommended. Without it, a method that does not match a base class method exactly would compile just fine and we’d never know that we made a mistake. Nothing else is required to override the base class method except to define a derived class method with the exact same signature (method name, parameter types, and parameter order).Note that we added a line to the new
onMousePressed(…)
method. We invoked the base class method with the same signature. This means we are augmenting the base class method with this override rather than replacing it. Overridable shape event handler methods perform core behaviors that make the event system work properly. Because we are augmenting the base class method and not simply replacing it, we want to ensure that all the default behavior is executed. We only want to add a toggle()
method invocation. Invoking the base class method using super
and relaying parameter values ensures that all default behavior will be executed as well. See Figure 9.3.2 for examples of the new program in action.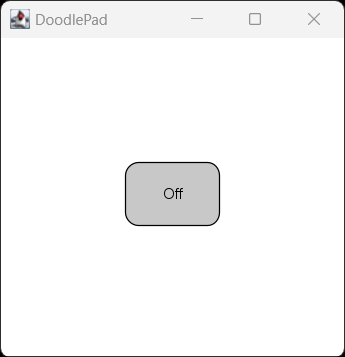
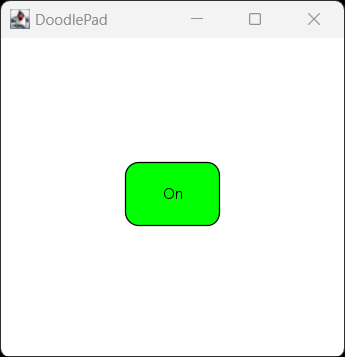
ToggleButton.java
(version 2). Off and On.That’s pretty good. It is a small upgrade to the previous version, but was it worth the effort? Looking at Figure 9.3.2, it seems that the button text is a bit small. We’d like to increase the font size. No problem! Because the button is really just an extension of a RoundRect, it inherits all of the RoundRect behavior, including the ability to adjust font size. We can simply add the appropriate methods to our
main(…)
method, as follows.public static void main(String[] args) {
// Create a new ToggleButton
ToggleButton myButton = new ToggleButton(100, 100, 75, 50);
myButton.setFontSize(24); // Change font size and style
myButton.setFontStyle(1);
}
The ToggleButton does not implement
setFontSize(…)
and setFontStyle(…)
, but it does inherit these methods from its base class. Modifying main(…)
and rerunning our program, we see the updated output in Figure 9.3.4. This would have taken some extra work had we stuck with our old implementation. We would have had to implement all new methods that modified the encapsulated RoundRect object. With inheritance, we get the behavior automatically; no modifications required.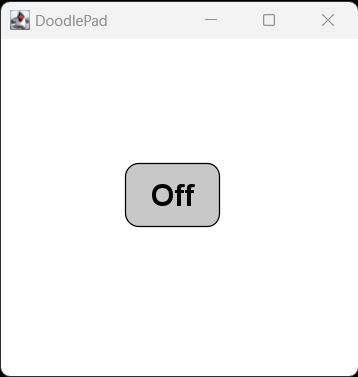
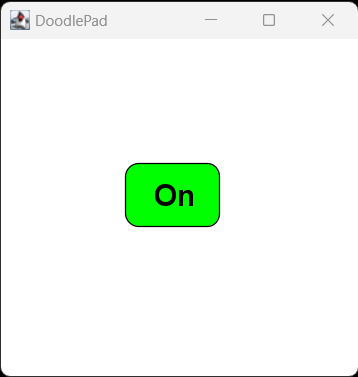
ToggleButton.java
(version 3). Off and On.In addition to overriding the
onMousePressed()
method, classes that extend Shape implement several additional methods that may be overridden to handle mouse-related events. See Appendix D for a list and more detail.