Section 14.10 Sprites
A core element of many classic computer games and other computer displays are animated characters or other objects called sprites. Sprites appear animated by displaying in a single place the sequential static frames of an animation loop, as you might see with an animated cartoon or flip book. Typical sprite animation loops show characters running, jumping, rotating, or other repetitive activities.
The frames of a sprite animation are stored together in a single image called a sprite sheet. A sprite display engine copies successive frames from the sprite sheet image and displays them in sequence at a given rate to create the sprite animation. The following image is a sprite sheet that may be used by the DoodlePad Sprite class. All five frames of the sprite animation stored in the following sprite sheet, where each frame is separated at a horizontal interval of 190 pixels.

Subsection 14.10.1 Sprite Constructors
To operate properly, Sprite objects need several items. They must load their sprite sheet images and have the parameters necessary for copying frames from the sprite sheet including frame width and the number of frames stored on the sprite sheet image. In addition, the coordinates for where the frames should be drawn must also be known, and optionally the dimensions of the frames. The following Sprite constructors are two of the most common and include the necessary parameters.
Constructor Signature | Description |
---|---|
Sprite(java.lang.String path, double x, double y, int frameWidth, int nFrames) 2 | Construct a new Sprite specifying a path to the sprite sheet image, the (x, y) coordinates indicating where to render the sprite, the width of a single frame, and the number of frames on the sprite sheet organized horizontally. |
Sprite(java.lang.String path, double x, double y, double frameWidth, int nFrames, int targetWidth, int targetHeight) 3 | Construct a new Sprite specifying a path to the sprite sheet image, the (x, y) coordinates indicating where to render the sprite, the width of a single frame, the number of frames on the sprite sheet organized horizontally, and the target dimensions for the sprite when frames are rendered. |
Subsection 14.10.2 Image Methods
As a subclass of Shape, Sprite objects inherit all the standard Shape methods. The primary additional method unique to the Sprite class is advance(). This is the method that instructs a Sprite object to move to the next frame in its animation sequence. The next frame image from the sprite sheet is copied and drawn at the Sprite location, possibly resizing to fit within target dimensions.
Method Signature | Description |
---|---|
public void advance() 4 | Advance sprite frame and repaint new frame image. |
Subsection 14.10.3 Flying Bird Example
The following example program creates a flying bird Sprite object that makes use of the sprite sheet displayed above. On the right is an animated image showing the flying bird Sprite in action. The Pad object’s timer functionality is used to drive the Sprite animation by invoking its advance() method repeatedly at a regular interval.
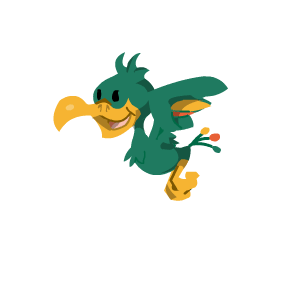
// FlyingBird.java
import doodlepad.*;
public class FlyingBird {
public static void main(String[] args) {
// Create a Pad and a Sprite
Pad pad = new Pad(300, 300);
Sprite sprite = new Sprite("FlyingBird.png", 50, 50, 190, 5);
// Set Pad timer with a Java lambda function that advances sprite
pad.setTickHandler( (Pad p, long when) -> { sprite.advance(); } );
pad.setTickRate(10);
pad.startTimer();
}
}
FlyingBird.java
www.greeniguanastudios.com/
doodlepad.org/dist/javadoc/doodlepad/Sprite.html#Sprite-java.lang.String-double-double-int-int-
doodlepad.org/dist/javadoc/doodlepad/Sprite.html#Sprite-java.lang.String-double-double-double-int-int-int-
doodlepad.org/dist/javadoc/doodlepad/Sprite.html#advance--