Section 6.2 do-while Statements
A third option for iteration in Java is the do-while statement. do-while is very similar to the while-statement, with one very important difference: the test for continuation is performed at the end of the code block, not the beginning, which is the way it works with the while-statement. Have a look at the following figure which illustrates the parts of a do-while statement.
One implication of the do-while continuation condition occuring at the end of the code block is that the block statements execute at least once before the condition is tested. Compare this to both the while-statement and the for-statement where the condition is tested before the code block. Consequently, with while- and for-statements, the code block may never execute. By contrast, the code block of a do-while always executes at least once.
This feature of the do-while statement makes it ideal for certain situations, such as when building a text menu for the terminal. With a text menu, we always want to print the menu options at least once before prompting the user for a response. Otherwise how will the user know how to answer?
Lastly, note that the
break
and continue
statements may be used with a do-while, in a manner similar to the for- and while-statements.
Listing 6.2.2 is a small program that demonstrates how to set up and run a text menu using a do-while statement. The loop continues while the response is not 0, which signals that the program should end. When the user enters a response of 0, the do-while statement breaks and the
System.exit(0)
command executes, which terminates the program.// DoWhileMenu.java
import java.util.Random;
import java.util.Scanner;
import doodlepad.*;
public class DoWhileMenu {
public static void main(String[] args) {
Scanner scn = new Scanner(System.in); // Helpers
Random rnd = new Random();
int r, g, b, ans = 0;
do { // Print menu
System.out.println("Choose a Shape to Create");
System.out.println("1. Oval");
System.out.println("2. Rectangle");
System.out.println("3. RoundRect");
System.out.println("0. Exit");
System.out.print("Enter a number: ");
ans = scn.nextInt(); // Prompt user
r = rnd.nextInt(256); // Random color
g = rnd.nextInt(256);
b = rnd.nextInt(256);
if (ans == 1) { // Take action
Oval o = new Oval();
o.setFillColor(r, g, b);
}
else if (ans == 2) {
Rectangle r1 = new Rectangle();
r1.setFillColor(r, g, b);
}
else if (ans == 3) {
RoundRect r2 = new RoundRect();
r2.setFillColor(r, g, b);
}
} while (ans != 0); // Keep going until exit
System.out.println("Goodbye");
System.exit(0); // Quit program
}
}
DoWhileMenu.java
Following is an sample session showing the menu in action. The output window generated is shown on the right.
javac -cp doodlepad.jar DoWhileMenu.java java -cp .;doodlepad.jar DoWhileMenu Choose a Shape to Create 1. Oval 2. Rectangle 3. RoundRect 0. Exit Enter a number: 1 Choose a Shape to Create 1. Oval 2. Rectangle 3. RoundRect 0. Exit Enter a number: 2 Choose a Shape to Create 1. Oval 2. Rectangle 3. RoundRect 0. Exit Enter a number: 3 Choose a Shape to Create 1. Oval 2. Rectangle 3. RoundRect 0. Exit Enter a number: 0 Goodbye
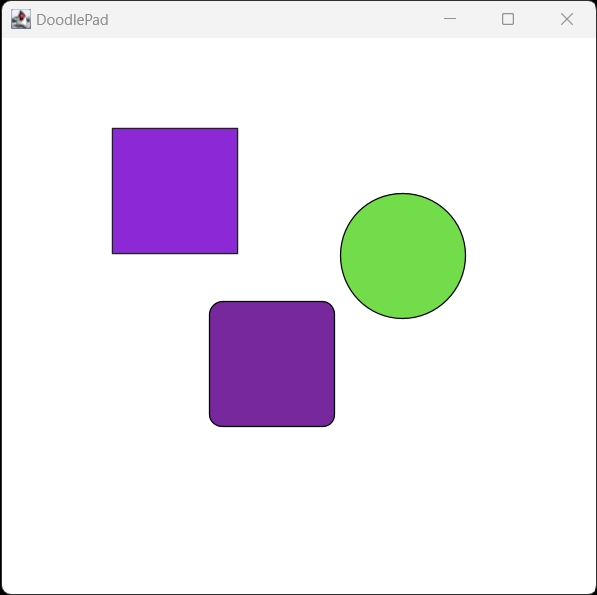
DoWhileMenu.java