Section 5.5 Collection Classes
String
, Random
, Scanner
, and StringBuilder
. The Java Core Library includes hundreds of classes that are available for you to use to solve your computational problems. One category of classes in the core library are called Collections. A Collection object is a kind of object that can hold and manage many other objects. In this section we will explore several of the most used Collection classes in Java.Subsection 5.5.1 ArrayList
The first Collection class we’ll explore is the ArrayList. An ArrayList is an object that holds references to zero or more other objects in an ordered sequence. An ArrayList grows or shrinks as needed. It is not necessary to select the size of an ArrayList before instantiation.
When an ArrayList is instantiated, it must specify the class of objects that it will hold. Because Java is a strongly typed language, we cannot freely assign just any object to a variable that is declared to hold another type of object. Despite this property, an ArrayList can be configured to hold objects of any class. The reason this is possible is because the ArrayList class is defined as what’s know as a generic. In short, the ArrayList is more of a class template that substitutes a class named as part of its constructor. A class is specified as a part of its constructor using the diamond operator (
< … >
).For example, if we want to instantiate an ArrayList that holds Oval objects, when writing the variable type declaration we must follow the ArrayList class name in the constructor with the diamond operator containing
Oval
. Following is an example of declaring and initializing an ArrayList to hold Oval objects.ArrayList<Oval> ovals = new ArrayList<>();
Note that the type used to declare the variable
ovals
is ArrayList<Oval>
, but the constructor used to create a new object to initialize the variable, is ArrayList<>
, having no class name in the diamond operator. Java knows that the variable is of type ArrayList<Oval>
and so it is not necessary to repeat the Oval
class in the constructor’s diamond operator.Subsection 5.5.2 ArrayList Methods
As is the case with all classes, ArrayList implements constructors and methods that allow us to instantiate and work with the object. Because the ArrayList class is designed to manage a series of objects, its methods relate to these management tasks as well. The following table lists many common ArrayList constructors and methods. The class of objects held by the ArrayList is not known until the ArrayList is instantiated. This class is indicated in the following table by a
T
for type. Once an ArrayList is instantiated, substitute T
with the class specified within the diamond operator.Method/Constructor | Returns | Description |
---|---|---|
new ArrayList<T>() |
--- |
Creates a new ArrayList with elements of type T. |
add(T element) |
boolean |
Add element to the end of the ArrayList. ͏ |
add(int idx, T element) |
void |
Inserts element at position idx, shifting objects down. |
get(int idx) |
T |
Returns a reference to the element at index idx . |
remove(int idx) |
T |
Removes and returns a reference to the item at index idx . |
size() |
int |
Returns the number of elements in the ArrayList. |
clear() |
void |
Removes all elements of the ArrayList, leaving it empty. |
contains(T element) |
boolean |
Returns true if the ArrayListcontains the element. |
indexOf(T element) |
int |
Returns the index of the first object matching element. |
Subsection 5.5.3 Filling ArrayLists and Accessing Elements
We can use what we know about iteration and our new ArrayList methods to fill an ArrayList with Oval objects. The following code snippet creates 20 Oval objects using the default Oval constructor and stores references to each in an ArrayList. Note that we are using the
add(…)
method of the ArrayList to add each new Oval object reference after the object is created.Oval o; // Helper variable and new ArrayList
ArrayList<Oval> ovals = new ArrayList<>();
// While loop iterating 20 times
int i = 0;
while ( i < 20 ) {
o = new Oval(); // Instantiate Oval
ovals.add( o ); // Store Oval in ArrayList
i++;
}
Without a data structure like ArrayList, we would have had to declare 20 variables to hold each Oval object reference, and then instantiate and assign each in order. Furthermore, we would have not been able to use the while-statement. That would have been tedious, at best. Instantiating 1000 Ovals would have been impractical.
Using a similar approach, we can access each Oval object. For example, if we wanted to change the fill color for each Oval, we could execute a code snippet like the following.
// While loop iterating over all elements
int i = 0;
while ( i < ovals.size() ) {
o = ovals.get(i); // Get reference to Oval at index i
o.setFillColor(255, 0, 0); // Set its fill color
i++;
}
In the above, we use the ArrayList’s
get(…)
method to get a reference to the Oval object at index i
and assign the reference to the temporary helper variable. Then we use the helper variable to access the object and invoke its setFillColor(…)
method.When we set up the while-statement condition we did not enter 20 explicitly. Instead, we asked the ArrayList its size by invoking the
size()
method. In general, it is a better idea to avoid using fixed parameters in favor of looking up parameter values. Later, if we decide that we do want 1000 Ovals, we will not have to hunt down and fix all the places in our code where we entered 20 explicitly.Subsection 5.5.4 Working with an ArrayList
Let’s bring a number of skills together in a larger program. In the following
RandomOvals.java
example, we are applying several skills, including:- Importing classes, both from the Java Core Library and a JAR file
- Naming our top-level class and matching to file name
- Declaring static variables in the outer class scope so the entire program has access
- Starting execution at
main(…)
- Defining a helper method for abstraction and reuse
- Instantiating new objects like Random and Oval
- Generating random numbers in a defined range
- Constructing a while-statement with an index that is used both to count iterations as well as to access ArrayList elements
- Invoking ArrayList methods like
add(…)
,get(…)
andsize()
- Invoking Oval methods like
setFillColor(…)
andsetMousePressedHandler(…)
- Declaring methods with well-defined signatures and handing mouse events
// RandomOvals.java
import java.util.ArrayList; // Import classes
import java.util.Random;
import doodlepad.*; // Import DoodlePad classes
public class RandomOvals { // public class
// Declare ArrayList of Ovals
public static ArrayList<Oval> ovals = new ArrayList<>();
// Start of execution
public static void main(String[] args) {
Oval o; // Helper variable
// Fill ArrayList with 20 randomly placed Oval objects
// Use a while to iterate 20 times
int i = 0; // Init counter
while (i < 20) { // While-statement
o = new Oval(); // Create an Oval object
o.setMousePressedHandler( RandomOvals::recolor );
ovals.add( o ); // Add Oval to ArrayList
i++; // Increment counter
}
}
// Event handler method that executes when an Oval is clicked
public static void recolor(Shape shp, double x, double y, int button) {
int r, g, b; // Helper variables
Oval o;
Random rnd = new Random(); // Declare a Random object
// Loop over all Oval objects and reset to a random fill color
int i = 0; // Init counter
while ( i < ovals.size() ) { // While loop
o = ovals.get(i); // Get the Oval at index i
r = rnd.nextInt(256); // Generate random color components
g = rnd.nextInt(256);
b = rnd.nextInt(256);
o.setFillColor(r, g, b); // Change fill color
i++; // Increment counter
}
}
}
RandomOvals.java
At the top of Listing 5.5.2 the program starts by importing all classes required that are not available by default. In this program we import the
ArrayList
and Random
classes from the java.util
package of Java core library as well as all DoodlePad classes.The main
RandomOvals
class contains two methods, the main(…)
method where execution starts, as well as a helper method named recolor
to be invoked when Oval objects are clicked.In the
main(…)
method we declare a helper variable and then set up a while-statement to help us create new Oval objects and fill the ArrayList. The loop counter i
is initialized to 0 and incremented at the end of the statement code block. The conditional expression ensures that the code block repeats 20 times only.Within the while-statement code block, we create a new Oval object and assign a reference to the helper variable. Then we use this variable to both assign a method to be invoked when the object is clicked, and to add the object to the end of the ArrayList.
The modifiers of the
recolor(…)
method are the same as main(…)
, public static void
. The signature is the one required to match an event handler method in DoodlePad. Within the method we start with helper variables and instantiate a Random object. Once again, we set up a while-statement to loop over all elements of the ArrayList. The condition does not specify the size of the ArrayList explicitly, but instead asks the ArrayList for the number of elements using its size()
method.Within the while-statement code block, we use the ArrayList
get(i)
method to get a reference to the object at index i
. Note that i
is used both as the loop counter as well as the index of each object in the ArrayList — a dual purpose. Following this we generate three integers in the proper range for the color elements: red, green, and blue, and then use them to set the Oval object’s fill to a random color. This repeats for all Ovals in the ArrayList.The figure on the right shows the program in action, after clicking one of the Oval objects. The shell session for compiling and running is below (for Windows Command Prompt). Make sure you have a copy of the
doodlepad.jar
file in your project folder and that you are using the correct syntax for your shell to compile and launch the program.javac -cp .;doodlepad.jar RandomOvals.java java -cp .;doodlepad.jar RandomOvals
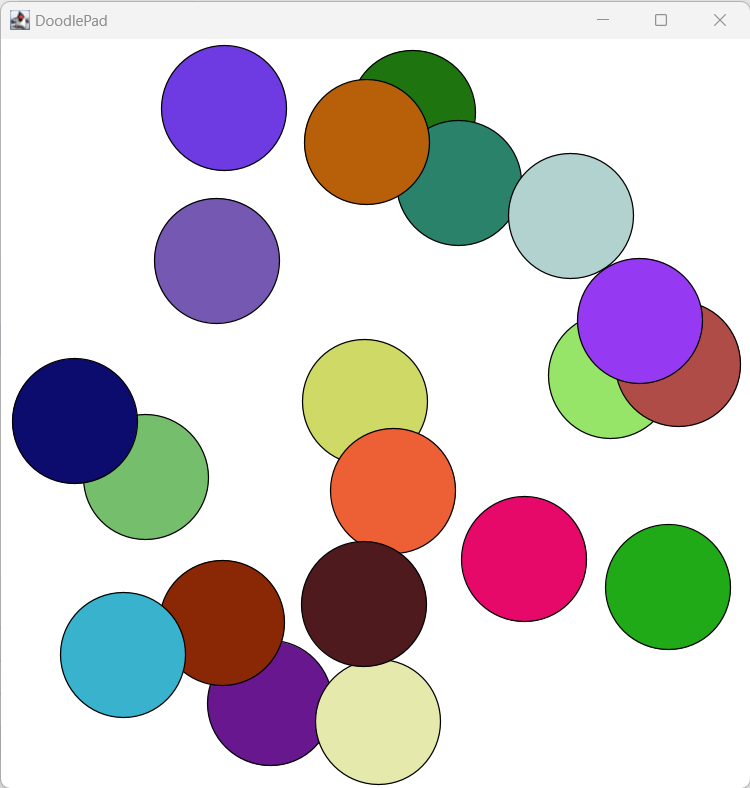
RandomOvals.java
Subsection 5.5.5 Autoboxing and Unboxing
A limitation of ArrayList is that it can hold object references only. But, as we know, primitive types are not reference types, and certainly we want the option to store collections of primitives like
int
and double
. How might we do that?To address this clear limitation, Java provides wrapper classes that allow us to wrap each primitive value in an object. With wrapper classes we are able to wrap primitives values before storing them in an ArrayList or other Collection. Java defines one wrapper class for each of the eight primitives. See Table 5.5.4. In general, the name of the wrapper class is the full primitive name starting with a capital letter.
Primitive type | Wrapper class |
---|---|
byte |
Byte |
short |
Short |
int |
Integer |
long |
Long |
float |
Float |
double |
Double |
boolean |
Boolean |
char |
Character |
This makes it possible to wrap and and store primitives in an ArrayList, but it is not convenient. Constantly wrapping and unwrapping primitives can become tedious, and quickly.
Fortnately, Java handles this for us by autoboxing and unboxing. Java automatically wraps, or "boxes", primitive types whenever necessary to place them in a collection like an ArrayList. It automatically unboxes primitive types when removing them from an ArrayList. We need only to remember to specify the wrapper class name in the diamond operator when declaring the ArrayList, and Java handles the rest.
In the following code snippet, we declare and fill an ArrayList<Integer> with 100 randomly generated integers. Once filled, we loop over the ArrayList<Integer> again, get each
int
at each successive index and print it. In both cases (adding and getting) we do not explicitly box or unbox the primitive type int
. Java automatically boxes each int
in an Integer
object before adding to the ArrayList, and unboxes the Integer
to an int
when getting and assigning to the primitive variable.Random rnd = new Random(); // New Random and ArrayList objects
ArrayList<Integer> ints = new ArrayList<>();
// Fill ArrayList with 1000 random ints
// The int is not boxed in an Integer class before being added
// Java takes care of this for us
int i = 0; // Counter
while (i < 1000) { // Loop
ints.add( rnd.nextInt(100) ); // Add int to ArrayList
i++; // Increment counter
}
// Get all ints and print
// Notice how val is assigned without unboxing Integer object
int val; // Helper variable
i = 0; // Reset counter
while (i < ints.size()) { // Loop
val = ints.get( i ); // Get int
System.out.println( val ); // Print int
i++; // Increment counter
}
Copy, compile and run Listing 5.5.5 to prove to yourself that this program works without error.
Subsection 5.5.6 HashSet
A second useful collection class provided by the Core Library is the HashSet. Like an ArrayList, a HashSet is a generic in the sense that when a new HashSet is declared and instantiated, the diamond operator is used to indicate the class of the objects that will be stored.
Two important behaviors of a HashSet are:
- duplicate values are not added to a HashSet
- the order of items added to a HashSet is not preserved
Just like a set in mathematics does not contain duplicates, a HashSet also does not hold duplicates. You may add the same element to a HashSet multiple times, but when you look at its contents, you will find only one occurrence. Table 5.5.6 lists several useful HashSet constructors and methods.
Method/Constructor | Returns | Description |
---|---|---|
new HashSet<T>() |
--- |
Creates a new HashSet with elements of type T. |
add(T element) |
boolean |
Add element to the HashSet, if not already present. |
remove(T object) |
T |
Removes and returns the object. ͏ |
size() |
int |
Returns the number of elements in the HashSet. |
clear() |
void |
Removes all elements of the HashSet, leaving it empty. |
A HashSet can be used to reduce a larger collection containing duplicate elements to a set of unique elements. In Listing 5.5.7 we instantiate a new HashSet<Character> and add 9
char
s to the HashSet. Afterwards we print the size of the HashSet to check that the duplicate values are not been stored. Remember that char
is a primitive type and so we declare the HashSet collection class to hold the wrapper class Character. Nevertheless, Java boxes our char
s automatically before adding them to the HashSet.// Unique.java
import java.util.HashSet; // Import class
public class Unique {
public static void main(String[] args) {
// Create a HashSet to hold Character objects
HashSet<Character> letters = new HashSet<>();
// Add letters as chars, automatically boxed
letters.add( 'D' );
letters.add( 'O' );
letters.add( 'G' );
letters.add( 'D' );
letters.add( 'I' );
letters.add( 'G' );
letters.add( 'G' );
letters.add( 'E' );
letters.add( 'R' );
System.out.println( letters.size() + " unique characters");
}
}
Unique.java
javac Unique.java java Unique 6 unique characters
Subsection 5.5.7 HashMap
A HashMap is the third Java Core Library collection class that we will introduce. Like an ArrayList, a HashMap is a sequential collection. Unlike an ArrayList, objects in a HashMap are keyed by another object, not an integer index. This can be an extremely useful capability, to map one object to another, independent of order. Think of a HashMap as a sequence of key-value pairs, where the key and value can be of any class type. To look up or set a value in a HashMap, provide the key value. Note, for a HashMap to work properly, it must be the case that the set of all keys are unique. Indeed, one method of a HashMap returns all keys. It won’t surprise you that a HashMap’s keys are returned as a set. Table 5.5.8 lists several useful HashMap constructors and methods.
Method/Constructor | Returns | Description |
---|---|---|
new HashMap<K><V>() |
--- |
Creates a new HashMap with key type K and value type V. |
put(K key, V value) |
V |
Associates the specified value with the specified key in this HashMap. |
get(K key) |
V |
Returns the value to which the specified key is associated, or null if this mapcontains no mapping for the key. |
remove(K key) |
K |
Removes and returns the value associated with the key. |
size() |
int |
Returns the number of elements in the HashMap. |
clear() |
void |
Removes all elements of the HashMap, leaving it empty. |
keySet() |
Set<K> |
Returns a set of all HashMap key values. ͏ |
Let’s say we wanted to write a program that stores the dinner orders of a table full of people. We may not know how many people will sit at a table, and an integer index is not be suitable to track orders because table chairs are not numbered, and even if they were, people may rearrange themselves. What we really want to do is to map a person’s name, a String, to a list of the items in their dinner order, perhaps an ArrayList<String>. That’s right; an ArrayList is an object, so we can declare our HashMap to be keyed by String objects, each of which is associated with its own ArrayList<String> value.
Take a moment to carefully study Listing 5.5.9. Here are some things to note.
- The HashMap
order
is keyed by String objects that relate to ArrayList<String> values. - We use the
get(…)
method with a String name key to get the ArrayList holding a particular customer, order and then modify it directly, as needed. - We can chain methods using multiple dot-operators, where intermediate objects are never assigned to variables, but stored temporarily as an automatic.
// Musketeers.java
// The Three Musketeers order dinner
import java.util.ArrayList;
import java.util.HashMap;
public class Musketeers {
public static void main(String[] args) {
// The Three Musketeers enter the restaurant: Athos, Porthos and Aramis
// Get ready to take their order.
HashMap<String, ArrayList<String>> order =
new HashMap<String, ArrayList<String>>();
// Add a new element for each Musketeer
order.put("Athos", new ArrayList<String>());
order.put("Porthos", new ArrayList<String>());
order.put("Aramis", new ArrayList<String>());
// Athos: I'll have a hamburger
order.get("Athos").add("Hamburger"); // Get Athos' order and add
// Porthos: Cheese fries please
order.get("Porthos").add("Cheese fries"); // Get Porthos' order and add
// Aramis: Greek Salad
order.get("Aramis").add("Greek salad"); // Get Aramis' order and add
// Athos: Nevermind. I'll have the Cobb salad
order.get("Athos").clear(); // Clear Athos' order
order.get("Athos").add("Cobb salad"); // Add a Cobb salad
// Porthos: Tuna sandwich as well
order.get("Porthos").add("Tuna sandwich");
// Aramis: Iced tea too
order.get("Aramis").add("Iced tea");
// Athos: Grape juice for me
order.get("Athos").add("Grape juice");
// Porthos: Please add a chocolate shake to my order
order.get("Porthos").add("Chocolate shake");
// Waiter recounts order
System.out.println("Athos:");
for (int i=0; i<order.get("Athos").size(); i++) {
System.out.println(" " + order.get("Athos").get(i));
}
System.out.println("Porthos:");
for (int i=0; i<order.get("Porthos").size(); i++) {
System.out.println(" " + order.get("Porthos").get(i));
}
System.out.println("Aramis:");
for (int i=0; i<order.get("Aramis").size(); i++) {
System.out.println(" " + order.get("Aramis").get(i));
}
}
}
Musketeers.java
javac Musketeers.java java Musketeers Athos: Cobb salad Grape juice Porthos: Cheese fries Tuna sandwich Chocolate shake Aramis: Greek salad Iced tea
You may have noticed that we did not demonstrate a way to iterate over the elements of a HashSet or a HashMap. Java provides easy ways to iterate over elements of these collections, but we defer that discussion to Section 6.3 when we introduce the for-each statement.