Section 14.1 DoodlePad Library
Below you’ll find a complete Java program that uses the DoodlePad library to create a graphics window (encapsulated as a
Pad
object) and instantiate an Oval
object. Once you compile and run your program, you will see a window like that shown in the figure on the right. This simple program demonstrates how DoodlePad removes the detail of the underlying windowing libraries allowing you to focus on learning Java and object oriented programming. Other than the include
statement added to the top of the program, we simply instantiated an Oval
object, and the rest was handled automatically.// MyFirstOval.java
import doodlepad.*;
public class MyFirstOval {
public static void main(String[] args) {
Oval oval1 = new Oval();
}
}
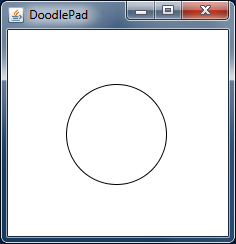
As we’ve seen, the
import
statement is necessary to load classes from external libraries that are not included in Java by default. The DoodlePad library (certainly) is not distributed with the JDK and therefore must be downloaded separately. Fortunately, this library is distributed as a single file named doodlepad.jar
1 and is freely available for you to download and use. The file extension JAR
is a clever shorthand for the term Java ARchive. In fact, a JAR
file is just a zip archive. Go ahead and use your favorite zip utility to open the file and peer into its contents. What you’ll see is a list of .class
files, which we know are compiled Java files. The doodlepad.jar
file is just a zip archive of precompiled Java classes.Unlike the classes that are included in the Java Platform, when compiling and running a program that uses DoodlePad classes, you must tell Java where to find them on your filesystem. That is, you must tell Java where to look for the
doodlepad.jar
file. Once it knows where to find these classes, the Java compiler will compile your program and check that you are using these classes in accordance with their exposed public interfaces. The Java runtime will bring it all to life as a running program.Most editors and Java programming environments provide ways to create projects that reference libraries so compiling and running is convenient. Here we will not use any special editor. Instead we use the commandline approach provided by the JDK shell commands to demonstrate how to compile and run programs that make use of the DoodlePad class library. We want to demonstrate how to use the library independent of any specific editor.
So, our core goal is to tell Java where to find our class libraries (i.e. our
.class
and .jar
files). Both the javac
command and the java
command allow the addition of several options when executed. We want to use the --class-path
option which informs the command which file system path to search for class libraries. This option can be shortened to -cp
for convenience.You may want to store all your
JAR
files in a common location on your file system, or take some other organizational approach. In the following example, we assume you have downloaded the doodlepad.jar
2 file and saved it to the same folder as your Java program. One word of caution. Many web browers consider JAR
files to be dangerous, and rightly so. They contain executable code. Your browser may refuse to download the doodlepad.jar
file until you give special permission to do so. This file is not executable. It contains only libraries to be used by other programs.Let’s assume you want to compile and run the
MyFirstOval.java
program given above. You already have the following two files in your folder: MyFirstOval.java
and doodlepad.jar
. Open a terminal window running your shell program and make sure the current directory is set to the folder containing your files. To compile your program execute the following command.javac -cp doodlepad.jar MyFirstOval.java
If all goes well, the compilation will finish and you will have a
MyFirstOval.class
file in your folder. If you see errors, double-check that the files are in your folder and that your shell has the current directory set to the same folder.The syntax for running the program will depend upon your operating system and shell. The reason being that you will use a separator character in your Java command, and that character is different between Windows and macOS/Linux. On Windows, the separator character is semicolon (
;
) and on macOS and Linux it is colon (:
). In your java
command you want Java to look for classes both in the current folder, which is specified by the special .
character, as well as in the doodlepad.jar
file. The MyFirstOval.class
file is saved in the current folder while the DoodlePad class files are in the doodlepad.jar
archive. The command to run your program on macOS or Linux is as follows. Note the use of the :
character to separate the current folder path (.
) and the doodlepad.jar
archive. Also recall that the last argument is the class at which to start execution -- the class having a public static void main(String[] args)
static method implemented.java -cp .:doodlepad.jar MyFirstOval
On Windows using the Command Prompt, use the following command syntax, which is the same as above except the path separator is now
;
.java -cp .;doodlepad.jar MyFirstOval
If using PowerShell on Windows, note that
;
character is used by the PowerShell language to terminal statements. We must escape the ;
character to ensure that it is not used by PowerShell, but instead is passed through unmodified to Java. To escape any character in PowerShell, preceded the character with a backtick (`
). The syntax for running a Java program while specifying a class path in PowerShell is as follows. Note the use of the backtick (`
) to escape the semicolon character (;
).java -cp .`;doodlepad.jar MyFirstOval
If successful, you will see a window like that in Figure 14.1.1.
If you plan to use DoodlePad classes in your examples (which I strongly encourage), make sure you have these details mastered and that you are able to compile and run a DoodlePad program like
MyFirstOval.java
on the operating system and shell of your choice. There is quite a bit of fun to come while writing interactive graphics program that make use of the DoodlePad library.doodlepad.jar
doodlepad.jar