Section 14.8 Images
Subsection 14.8.1 Image Constructors
Images may be constructed blank, or upon construction they may load and display an image from a file. This behavior is governed by the constructor used to create an Image. Following are three Image object constructors. The first constructor creates an blank Image with the givel location (xm y) and dimensions (width, height). The second constructor creates an Image object and loads an image from a file, given a path to it on the file system. The dimensions of the Image are set to match the dimensions of the image loaded from the file. The third constructor creats an Image object of the given dimensions, and also loads and resizes the image stored in the given file path prior to displaying it. For all Image constructors, refer to the docs.
Constructor Signature | Description |
---|---|
public Image(double x, double y, double width, double height)β1β | An Image object constructor that creates a blank Image object with an upper left corner at (x,y) and dimensions (width, height). |
public Image(java.lang.String path, double x, double y)β2β | An Image object constructor that creates an Image object and loads and displays an image from the path given as the first argument. The upper left corner of the image is at (x,y) and its dimensions map the dimensions of the loaded image. Loadable Image formats include GIF, PNG, JPEG, BMP, and WBMP. |
public Image(java.lang.String path, double x, double y, double width, double height)β3β | An Image object constructor that creates an Image object and loads and displays an image from the path given as the first argument. The upper left corner of the image is at (x,y) and its dimensions (width, height). Loadable Image formats include GIF, PNG, JPEG, BMP, and WBMP. |
The following simple example demonstrates how to create a new Image object using the constructor that loads and displays an image upon construction. The screenshot on the right shows the result of the
Image1.java
program. While strictly not required, in this example an instance of the Pad object is created first to set the size of the initial window and to give it a title. The image is a selfie taken by the wild Indonesian macaque named Naturo who was the subject of a well-known copyright dispute.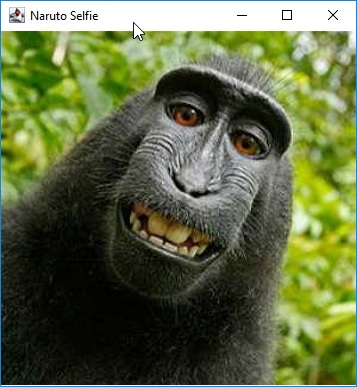
// Image1.java
import doodlepad.*;
public class Image1 {
public static void main(String[] args) {
Pad pad = new Pad("Naruto Selfie", 355, 355);
Image im = new Image("naruto-selfie.png", 0, 0);
}
}
Subsection 14.8.2 Image Methods
The Image object implements several methods for modifying its pixels, whether that be one pixel at at time, or by setting pixels to form shapes or text. Following are selected Image object methods with descriptions.
Method Signature | Description |
---|---|
public void setPixel(int x, int y, int red, int green, int blue)β4β | Set the color of the pixel at the coordinate (x, y) to the color identified by (red, green, blue). (See Colorsβ5β.) |
public int getRed(int x, int y)β6β | Return an int corresponding to the red component of the pixel color at (x, y). |
public int getGreen(int x, int y)β7β | Return an int corresponding to the green component of the pixel color at (x, y). |
public int getBlue(int x, int y)β8β | Return an int corresponding to the blue component of the pixel color at (x, y). |
public int getAlpha(int x, int y)β9β | Return an int corresponding to the alpha (transparency) component of the pixel color at (x, y). |
public void drawArc(double x, double y, double width, double height, double startAngle, double arcAngle)β10β | Draw an arc on the Image defined by a section of an ellipse bounded by the rectangle with upper left corner at (x, y) and size (width, height). The arc starts at angle startAngle (degrees) and extends by arcAngle (degrees). |
public void drawLine(double x1, double y1, double x2, double y2)β11β | Draw a line on the Image from the coordinate (x1, y1) to (x2, y2). |
public void drawOval(double x, double y, double width, double height)β12β | Draw an oval on the Image within the bounding box having an upper left corner at (x, y) and dimensions (width, height). |
public void drawPolygon(double[] xPoints, double[] yPoints)β13β | Draw a polygon on the Image that connects corresponding coordinate pairs stored in the two arrays xPoints and yPoints. |
public void drawPolygon(java.util.List<Point> points)β14β | Draw a polygon on the Image that connects coordinates stored as a List<> of Pointβ15β objects. |
public void drawRectangle(double x, double y, double width, double height)β16β | Draw a rectangle within the bounding box having an upper left corner at (x, y) and dimensions (width, height). |
public void drawRoundRect(double x, double y, double width, double height, double arcWidth, double arcHeight)β17β | Draw a rounded rectangle within the bounding box having an upper left corner at (x, y), dimensions (width, height), and rounded corners defined by an arc with width arcWidth and height arcHeight. |
public void drawText(java.lang.String text, double x, double y)β18β | Draw text on an Image stored in the String text, with an upper left corner defined by (x, y). |
public void drawText(java.lang.String text, double x, double y, int size)β19β | Draw text on an Image stored in the String text, with an upper left corner defined by (x, y), and a font size of size. |
You will note that none of the previous methods set the fill color, stroke color, or stroke width to be used when drawing on an Image. To set the fill and stroke properties of drawn lines and shapes, set these values using the Image objectβs methods prior to invoking one its drawing methods. Once set, these settings are used for all subsequent drawing performed on an Image object, until new settings are specified.
Method Signature | Description |
---|---|
public void setFillColor(double red, double green, double blue)β20β | Set the color to be used to fill shapes drawn on an Image. |
public void setStrokeColor(double red, double green, double blue)β21β | Set the color to be used to outline shapes drawn on an Image. |
public void setStrokeWidth(double width)β22β | Set the width in pixels of the stroke to outline any shape that is drawn on an Image. |
Subsection 14.8.3 A Simple Example
The following example program demonstrates the use of Image object methods used to draw on the Image. In this example 200 lines are drawn on the Image object using a for statement. Each line is drawn after setting random endpoint coordinates, random width, and random stroke color.
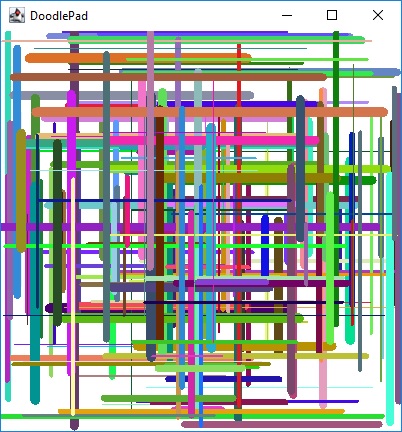
Image2.java
// Image2.java
import java.util.Random;
import doodlepad.*;
public class Image2 {
public static void main(String[] args) {
double x1, x2, y1, y2, w;
int r, g, b;
// Create a Pad object, an Image object for the Pad,
// and a Random object for generating random numbers.
Pad pad = new Pad(400, 400);
Image im = new Image(0, 0, 400, 400);
Random rnd = new Random();
for (int i=0; i<100; i++) {
// Random numbers
y1 = 400*rnd.nextDouble();
x1 = 200*rnd.nextDouble();
x2 = 200+200*rnd.nextDouble();
w = 10*rnd.nextDouble();
r = rnd.nextInt(256);
g = rnd.nextInt(256);
b = rnd.nextInt(256);
// Draw line on Image
im.setStrokeColor(r,g,b);
im.setStrokeWidth(w);
im.drawLine(x1,y1,x2,y1);
// More random numbers
x1 = 400*rnd.nextDouble();
y1 = 200*rnd.nextDouble();
y2 = 200+200*rnd.nextDouble();
w = 10*rnd.nextDouble();
r = rnd.nextInt(256);
g = rnd.nextInt(256);
b = rnd.nextInt(256);
// Draw line on Image
im.setStrokeColor(r,g,b);
im.setStrokeWidth(w);
im.drawLine(x1,y1,x1,y2);
}
}
}
Image2.java
Subsection 14.8.4 An Image as a Shape
Because Image is a subclass of Shape it inherits all of the Shape methods. For example, you may resize and move an Image as well as respond to all the standard mouse events. This gives you the ability to programmatically draw on the Image in response to interacting with it using the mouse. The following example draws a randomly-sized green oval where the mouse is pressed. For a more complete program that uses this technique to create a drawing program using DoodlePad, refer to the Interactive Drawing example.
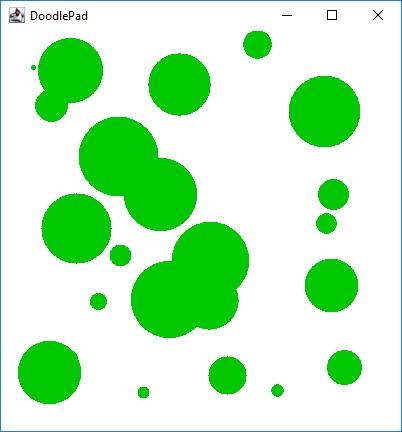
Image3.java
// Image3.java
import doodlepad.*;
public class Image3 {
public static void main(String[] args) {
// Create Pad and Image
Pad pad = new Pad(400, 400);
Image im = new Image(0, 0, 400, 400);
// Set up mouse event handler
im.setMousePressedHandler( Image3::onSpot );
// Set drawing fill color to green
im.setFillColor(0,200,0);
}
// What to do when mouse is pressed on Image
public static void onSpot(Shape shp, double cx, double cy, int button) {
// Cast Shape to Image
Image im = (Image)shp;
// Draw randomly sized oval
double radius = Math.random()*40;
im.drawOval(cx-radius, cy-radius, 2*radius, 2*radius);
}
}
Image3.java
doodlepad.org/dist/javadoc/doodlepad/Image.html#Image-double-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#Image-java.lang.String-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#Image-java.lang.String-double-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#setPixel-int-int-int-int-int-
doodlepad.org/colors.html
doodlepad.org/dist/javadoc/doodlepad/Image.html#getRed-int-int-
doodlepad.org/dist/javadoc/doodlepad/Image.html#getGreen-int-int-
doodlepad.org/dist/javadoc/doodlepad/Image.html#getBlue-int-int-
doodlepad.org/dist/javadoc/doodlepad/Image.html#getAlpha-int-int-
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawArc-double-double-double-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawLine-double-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#getAlpha-int-int-
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawPolygon-double:A-double:A-
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawPolygon-java.util.List-
doodlepad.org/dist/javadoc/doodlepad/Point.html
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawRectangle-double-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#getAlpha-int-int-
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawText-java.lang.String-double-double-
doodlepad.org/dist/javadoc/doodlepad/Image.html#drawText-java.lang.String-double-double-int-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setFillColor-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setStrokeColor-double-double-double-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setStrokeWidth-double-