Section 14.3 Creating Shapes
In Section 14.1 we created our first Oval object. We used the
Oval
class constructor that takes no arguments. every Shape in DoodlePad has a nullary (no-argument) constructor for convenience. For all Shapes, the nullary constructor chooses the initial location of the Shape to be random (using a Random
object) and sets the width and height to 100. Alternative Shape constructors let use set the location and size as parameters.DoodlePad defines a variety of graphic objects that you can instantiate and interact with, including basic shapes. Available basic shape classes include the following. Don’t miss other DoodlePad classes such as
Polygon
, Pad
, Path
, Image
, Text
, Sprite
and more.Shape | Description |
---|---|
Rectangle |
Your garden variety Rectangle |
Oval |
Another name for an Ellipse |
RoundRect |
A Rectangle with rounded corners |
Line |
A straight line between two points |
Arc |
A curved line that is part of an ellipse |
Subsection 14.3.1 Rectangle
Rectangles are one of the more simple shapes in DoodlePad. Creating a new Rectangle is as simple as creating an instance of the Rectangle class. The Rectangle class’s nullary constructor creates a 100 pixel × 100 pixel Rectangle at a randomly selected location, but other Rectangle constructors give you more control of the Rectangle created. For example, the following sample program creates a 70 pixel × 40 pixel Rectangle with its upper left corner at the location (50, 60).
// RectangleDemo1.java
import doodlepad.*;
public class RectangleDemo1 {
public static void main(String[] args) {
Rectangle r1 = new Rectangle(50, 60, 70, 40);
}
}
The Rectangle size and location are passed as numerical arguments to the Rectangle constructor. The first pair of arguments correspond to the Rectangle location (x=50, y=60) and the second pair correspond to its size (width=70, height=40). This is a pattern that is repeated in many graphic object constructors in DoodlePad.
Subsection 14.3.2 Oval
Creating an Oval is very similar to creating a Rectangle. An Oval’s nullary constructor creates a new 100 pixel × 100 pixel ellipse Shape with a randomly selected starting location. An Oval also has a four-argument constructor similar to a Rectangle. The first two arguments of this constructor are the x and y coordinates of the upper left corner of the Oval’s bounding box and the second two arguments are the Oval’s width and height. Note that the four numerical argument values of this Oval constructor can be thought of as describing the bounding box within which the Oval is be created.
The only difference between the following example program and the previous example is that the following sample program creates a 70 pixel × 40 pixel Oval with its upper left corner at the location (50, 60) isntead of a Rectangle.
// OvalDemo1.java
import doodlepad.*;
public class OvalDemo1 {
public static void main(String[] args) {
Oval r1 = new Oval(50, 60, 70, 40);
}
}
Subsection 14.3.3 RoundRect
The RoundRect class differs from Rectangle in that the corners of a RoundRect are, well, rounded. In addition to specifying the location and size of a RoundRect, you must also specify the width and height of the arcs that form the rounded corners. These additional parameters are called arcWidth and arcHeight and come after x-location, y-location, width and height in the RoundRect constructor.
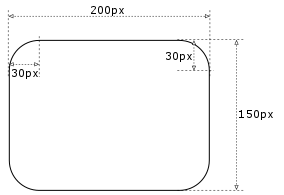
In the following example we create a RoundRect object at (10, 10) with width = 200, height = 150, arcWidth and arcHeight = 30. The diagram on the right illustrates how these parameters are used to define the shape. Note that the arcWidth and arcHeight parameters are not required to have equal values.
// RoundRectDemo1.java
import doodlepad.*;
public class RoundRectDemo1 {
public static void main(String[] args) {
RoundRect r1 = new RoundRect(10, 10, 200, 150, 30, 30);
}
}
Subsection 14.3.4 Arc
The Arc shape class can be thought of as a pie-section taken from an Oval. In fact, to define an Arc one starts with the location and dimensions of an Oval and then adds the start and angular extent of the section to be taken. In DoodlePad these angles are defined with 0° indicated by a horizontal line extending from the center of the oval to the right. As the angle increases the line rotates in the counter clockwise direction.
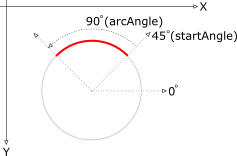
The beginning of an arc is specified with an angle between 0° and 360°, and the length of the arc is determined by an angle that the arc sweeps. The arc itself follows the outline of the equivalent oval. The figure on the right describes the arc specified (in red) by the program below. The first four arguments of the Arc constructor are the same as an Oval (x, y, width, height). The last two parameters are the starting angle (startAngle) and the extent of the arc (arcAngle) measure in degrees.
When an arc is filled, it becomes a pie section, which can be filled and outlined to form a pie-shaped graphic object. When an arc is not filled, as is the case with the example below, only the segment of the equivalent Oval’s outline is rendered.
// ArcDemo1.java
import doodlepad.*;
public class ArcDemo1 {
public static void main(String[] args) {
// Create the Arc starting at 45 degrees and extending for 90 degrees
Arc a1 = new Arc(100, 100, 200, 200, 45, 90);
// Do not fill the arc so only the arc is drawn.
a1.setFilled(false);
}
}
Subsection 14.3.5 Line
The Line shape is created by specifying its two endpoint coordinates. The Line constructor takes four values, (x1, y1, x2, y2), where (x1, y1) is the first endpoint and (x2, y2) is the second endpoint.
The example below creates a diagonal Line shape between the endpoints (100, 100) and (200, 200).
// LineDemo1.java
import doodlepad.*;
public class LineDemo1 {
public static void main(String[] args) {
// Create a diagonal Line shape from (100, 100) and (200, 200)
Line l1 = new Line(100, 100, 200, 200);
}
}
DoodlePad provides more ways to create Shape classes beyond those described in this chapter. If you’d like to skip ahead to investigate more complex techniques, have a look at Path Shapes 14.7, Polygon Shapes 7.6. Images 14.8, and Sprites 14.10.