Section 8.4 Shadowing
With nested scopes we can define a variable within a method or constructor scope and then another having the same name in an outer object or class scope. This doesn’t cause a problem until we want to access one of the two variables by name from the inner scope. An ambiguity arises. Java cannot tell if you intended to reference the variable declared within the inner scope or the outer scope. To solve this problem, Java always chooses a variable by searching for the variable declaration starting in the current scope, and if not found, it proceeds to outer scopes, progressively.
Subsection 8.4.1 Shadowing Troubles
Consider the
ClickThick.java
program source code in Listing 8.4.1. This program creates an initial Line shape object assigned to the variable line
, which is set up to invoke the object-scoped onPressed(…)
method when the mouse clicks the line. If the left mouse button is used to click the line, the thickness of the line is doubled. If the right mouse button is used to click the line, the thickness of the line is halved.// ClickThick.java
import doodlepad.*; // Import Doodlepad
// Classes
// Class that illustrates Line thickness
public class ClickThick {
private Line line; // Object-scoped variable
public ClickThick() { // Constructor
// Create Line object and assign to variable
Line line; // This variable SHADOWS
line = new Line(100, 100, 500, 100); // the line variable above
line.setStrokeWidth(10);
line.setMousePressedHandler( this::onPressed ); // Assign event handler
}
// Event handler method
public void onPressed(Shape shp, double x, double y, int button) {
double w = line.getStrokeWidth(); // Current line thickness
if (button == 1) { // Left mouse pressed
line.setStrokeWidth( 2*w ); // Double thickness
} else if (button == 3) { // Right mouse pressed
line.setStrokeWidth( w/2 ); // Halve thickness
}
}
// Create object
public static void main(String[] args) {
new ClickThick();
}
}
ClickThick.java
When we compile and run the program, the expected window appears (Figure 8.4.2). But when we click the line, the console prints an error to the terminal, the top several lines of which is in the session below. The main error reported is
java.lang.NullPointerException
because "this.line" is null (line 18). This error is telling us that the line
variable is null
so our attempt to get the line thickness using line.getStrokeWidth()
failed.javac -cp doodlepad.jar ClickThick.java java -cp .;doodlepad.jar ClickThick Exception in thread "AWT-EventQueue-0" java.lang.NullPointerException: Cannot invoke "doodlepad.Line.getStrokeWidth()" because "this.line" is null at ClickThick.onPressed(ClickThick.java:18) at doodlepad.Shape.onMousePressed(Shape.java:1402) at doodlepad.Shape$1.mousePressed(Shape.java:231) at doodlepad.Pad$7.mousePressed(Pad.java:454) ...
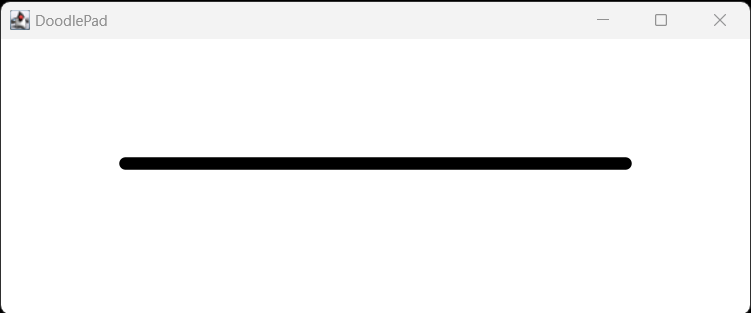
ClickThick.java
This might seem odd because it is clear that the
line
variable is initialized in the constructor, in the statement Line line = new Line(100, 100, 500, 100)
. So what could be wrong? Can you spot the error?If you look closely, you will see that there are two
line
variables. One is declared in the constructor and the other in the outer scope of the object. In the constructor, only the locally scoped line
variable is initialized. The other line
variable declared in the scope of the object is never initialized. When the constructor exits its scope is gone, and so is its locally scoped line
variable. When the Line object is clicked the onPressed(…)
method is invoked. There is no line
variable declared in this method and so the variable referenced is the one declared in the object scope, which still has the value of null
because it was never initialized. This is the source of the error. It was caused by mistakenly declaring a second line
variable in the scope of the constructor, which shadows the object-scoped line
variable.The intention was to initialize the object-scoped
line
variable in the constructor. Unfortunately, the local constructor-scoped line
variable shadows the object-scoped variable because it has the same name. The constructor initialized the wrong line
variable. See Figure 8.4.3 for an illustration of the situation. To solve the problem we can eliminate the declaration of the locally-scoped line
variable by removing the class name Line
, leaving only the initialization. Specifically, line = new Line(100, 100, 500, 100)
. After this modification, the program works correctly.line
This example illustrates how shadowing works, and how it can lead to trouble if you are not careful about where variables are declared and how they are initialized.
Subsection 8.4.2 Peering Around a Shadow
Even when an outer-scoped variable is shadowed by a inner-scoped variable declaration, the shadowed outer-scoped variable may be accessed directly by setting its scope explicitly. Recall that class-scoped variables and methods may be accessed using the class name and the dot-operator; think about
Math.sqrt(…)
. Also, the current object-scoped variable is set using the this
keyword and the dot-operator. These techniques may be used from an inner scope just as well as from an outer scope. For example, from within the ClickThick
constructor in Listing 8.4.1, to ensure that I was accessing the object-scoped line
variable and not the locally-scoped line
, I could have used this
to set the scope explicitly, this.line = new Line(100, 100, 500, 100)
. While Java will search progressively broader scopes for an identifier that matches one that you reference, explicit scoping using this
or a class name and the dot-operator removes any ambiguity.One common situation in which explicit object scoping is required is when defining a constructor that initializes instance variables having the same name as constructor parameters. It is convenient to assign names to constructor parameters that match instance variable names they are intended to initialize. Of course, these parameters shadow the instance variables, so explicit scoping using
this
is required.As a simple example, let’s assume my program includes a
Cat
class that tracks the cat’s name and age. The constructor is used to initialize these two instance variables. In Listing 8.4.4 you will see that the Cat
class defines the instance variables name
and age
, and the constructor takes the two parameters also declared as name
and age
. For the constructor to perform the assignment correctly, it must explicitly set the scope of the instance variables to the object using this
.// Cat.java
// Cat class
public class Cat {
private String name; // Instance variables
private int age;
// Constructor that initializes this instance
public Cat(String name, int age) {
this.name = name; // Avoids shadowing
this.age = age;
}
// ...
}
Cat.java
This pattern of using constructor parameters to initialize instance variables is common. The intentions are clear and the explicit scoping avoids any potential ambiguity.