Section 1.4 Object Oriented Programming
Object-oriented programming (OOP) is a programming paradigm centered around the idea of organizing and structuring a program as software objects that encapsulate data and behavior. In a way, these object are models of discrete elements that work together as part of a larger system designed to solve a particular problem.
Imagine that you have been called upon to write a program that manages the items in a brick-and-mortar library. Among other things, the job of a library is to maintain, track, and lend items from its collection, including books, reference materials, digital recordings, and more. It must also track who has permission to borrow items from the library. Stop and think for a moment about what might be the software objects associated that would make up a library management system?
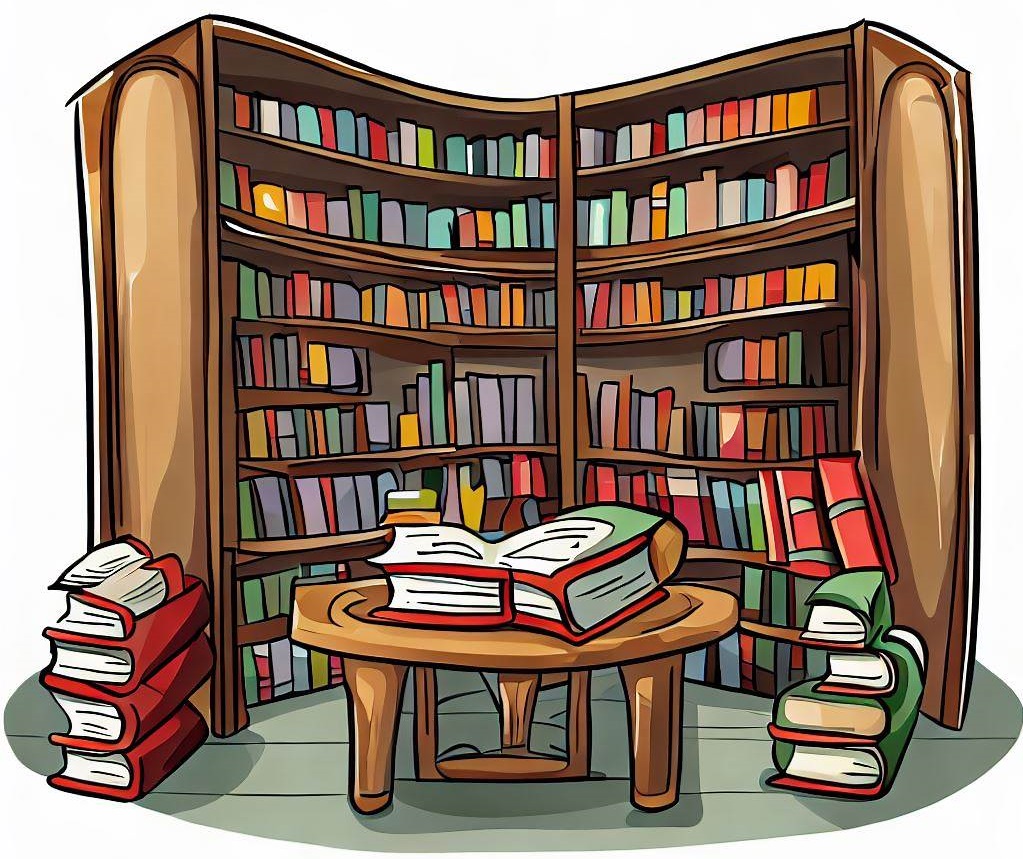
There are several physical items that we can identify right away. For example, a Book, a DVD, a Borrower, or even a Bookshelf, might be items that your library management software system might need to model and manage. A Book object may be described by several data items, including a title, authors, publication date, a Dewey Decimal identifier, library storage location, and whether or not it is currently checked out or available for circulation. Each of these data items are properties of a Book object and, in accordinate with the the principles of object oriented programming, should be encapsulated within the Book software object. The same arguments may be made for other library system objects, such as DVD, Borrower, and Bookshelf.
It is not necessary for objects to be physical. For example, a book Collection might itself be an object. A book Collection might have encapsulated properties such as the number of Book objects it contains, the name of the building to which the Collection belongs, who is responsible for the collection, and of course the Book objects themselves. A book Collection object may be able to do things, such as add a Book object to or remove a Book object from its collection and iterate over the collection, returning one Book object at a time.
Another example of an object might be the relationship between a Book object and a Borrower object. A Book may have had many Borrowers and a Borrower may be borrowing many Books. Rather than choose to encapsulate a list of Books in a Borrower or a list of Borrows in a Book, you might want an object that captures that relationship between a book and a borrower. We might encapsulate the properties of this relationship in a Circulate object. A Circulate object might have one reference to a Book, one to a Borrower, a date that the Book was lent, a date that the Book is due to be returned to the Library, and whether or not it is overdue.
Because we can define many types of objects (e.g. Book vs. Borrower), and there may be many instances of each kind, we must have a unique template for creating each type. In object oriented programming this template is called a class. Classes serve as blueprints for creating new objects. They define the data that each object instance can hold (e.g. title and author) as well as what it can do (e.g. add or remove a Book). We will learn how to do this ourselves in the chapter Custom Classes.
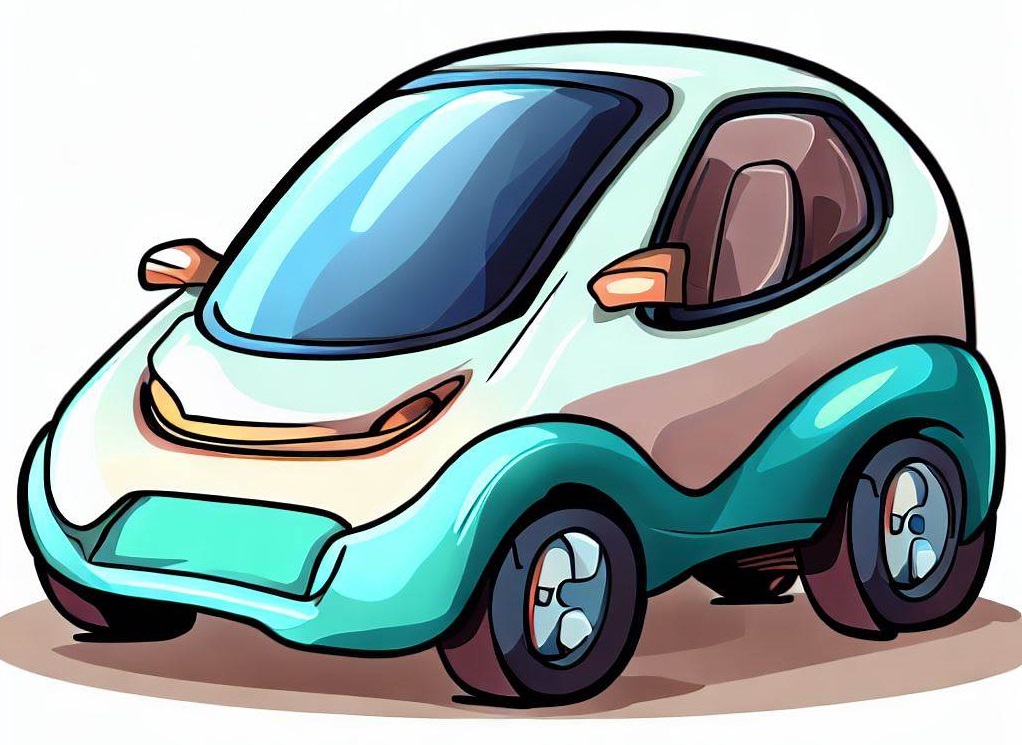
One of the real benefits of object oriented programming is abstraction. When I drive a car, I don’t need to know all the underlying technical details about how the car is able to move. I know that if I press the start button in my electric vehicle, the engine turns on. I know if I put the car in gear and press the accelerator pedal, the car moves. There is quite a lot of engineering that makes this possible, but I don’t need to know all the details.
From my perspective as a driver, I need only understand how to make the car move, turn, and stop. The details of how the car works have been abstracted away, making it possible for me to operate a car with a minimal amount of knowledge and skill. This is power of abstraction.
Let’s think again about our library Collection object. A Collection knows how to add a reference to a Book object so the Book is considered to be in the Collection. How does this work? What is involved with actually putting a Book object into a Collection object? If you are adding this behavior to the Collection class, then you’ll need to know the details. But if you are only using the behavior, then you can ignore the details and just invoke the behavior when you need it, very similar to driving a car. You can just ask your Collection object to add a Book object to itself. The details don’t matter (assuming you trust the author). They have been abstracted away. As we’ll learn, most modern programming languages come with prewritten and tested software libraries that we can use to write our programs. It is not necessary for us to learn the underlying details about how each works, only how to use them. The detail has been abstracted away and encapsulated by libraries of classes that we can use. Nice!
One more benefit of object oriented programning is inheritance. In most object oriented programming languages it is possible to relate classes to one another in a hierarchical fashion. For example, a Book class and a DVD class might both be related to a more general class called CirculationItem, which encapsualtes all the common properties and behavior shared by all items in a library that circulate. A Book object has its unique properties, like number of pages, and a DVD object has its unique properties, such as run duration, but both have properties like Decimal Dewey number or storage location. Rather than duplicate these common properties in each class, we can add them to the more general CirculationItem class, and then relate both Book and DVD to CirculationItem through inheritance. The inheritance relationship provides both Book and DVD with access to the properties and behaviors in the more general CirculationItem class. As programmers, this allows us to author these common properties and behaviors once, in CirculationItem, but give all inheriting classes access to the same properties and behaviors. Updating the behaviors in CirculationItem automatically shares these updates with both Book and DVD. When used correctly, inheritance can be very powerful.
From an object oriented programming standpoint, both Book and DVD are two kinds of CirculationItem. In that sense, Book and DVD are different forms of CirculationItem, and so it is possible to treat both as if they were CirculationItem classes. This is one way that object oriented programming supports another concept called polymorphism (many forms). We’ll learn more about inheritance in Chapter 9 and the many forms of polymorphism in object oriented programming throughout the remainder of this book.
Taken together, the key principles of object-oriented programming are:
- Encapsulation: Encapsulation refers to the bundling of data and related behavior (methods or functions) into a single unit called an object. The data, also known as attributes, fields, or properties, tend be accessed and modified by the methods defined in the class. Encapsulation helps in organizing code, as the internal workings of an object are hidden from the outside, and it also provides data protection and security.
- Inheritance: Inheritance allows the creation of new classes based on existing classes, inheriting their attributes and behaviors. The existing class is called the "parent class", "base class", or "superclass", and the new class is called the "child class","derived class", or "subclass". The child class may extend or modify the behavior of the parent class by adding new methods or overriding existing ones. Inheritance promotes code reuse and supports the concept of hierarchical relationships between classes.
- Polymorphism: Polymorphism means that objects of different classes can be treated as objects of a common superclass. It allows you to write code that can work with objects of different types (classes), as long as they share a common interface or base class. Polymorphism enables more flexible and extensible code, as it allows for method overriding and method overloading. Method overriding means that a subclass can provide its own implementation of a method defined in the superclass, while method overloading allows multiple methods with the same name but different parameters to coexist in a class.
- Abstraction: Abstraction focuses on capturing the essential features and behaviors of an object while hiding unnecessary details. It allows you to create abstract classes or interfaces that define a set of methods without specifying their implementation. Concrete classes, which inherit from abstract classes or implement interfaces, provide the implementation details. Abstraction helps in designing complex systems by breaking them down into manageable and modular components.
By applying these principles, object oriented programming promotes modular, reusable, and maintainable code. It allows you to model real-world entities or concepts as objects, which interact with each other to solve problems or simulate systems. OOP languages, such as Java, C++, and Python, provide constructs to support these principles and enable the creation of object oriented systems.
Don’t worry if these concept seem vague or confusing. They will become more clear as we explore examples of how each is implemented in Java and explain their benefits.