Section 14.6 Event-Driven Programming
Perhaps the most distinguishing feature of the DoodlePad library is the ease and extent to which it supports event driven programming. All DoodlePad Shape objects as well as the Pad object may be set up to invoke custom methods in response to a wide range of user interaction. For example, you may want the change the fill color of one or more shapes when the user hovers over the shape or clicks the shape with the mouse. Alternatively, you may want to move a Shape in specific direction when the user presses one of the arrow keys. The Pad object implements timer functionality that raises tick events at a user-specified rate. You may want to invoke one or more of your custom methods each time the Pad’s timer ticks, which may be accomplished using the Pad’s tick event.
By default, all Shape objects as well as Pad objects trigger a wide array of events. But sometimes you don’t want a Shape or Pad object to react to events at all, allowing all interactions to be handled by the Shape object or Pad object underneath. For example, imagine a Happy Face made up of a large yellow Oval for the face, two smaller black Ovals for the eyes and an Arc for the smile. You might want the eyes and smile to ignore all mouse interaction so that only the underlying yellow face Oval detects all mouse interaction and triggers mouse events. Another use case occurs when you want to create a button with a label by placing a Text Shape over a RoundRect Shape. In this case you want the Text to ignore all mouse interaction and allow the underlying RoundRect to respond to the mouse. This is easily accomplished by disabling events on your top-level Shapes. Use the setEventsEnabled(...) method to disable or re-enable event triggering for any Shape object or any Pad object.
This ability to respond to mouse, keyboard, timer and other events allows DoodlePad to be used to develop a wide range of fun interactive graphics programs that are limited only by your imagination.
Subsection 14.6.1 Connecting Events and Methods with Method References
The simplest way to invoke a method in response to an event is to connect the event associated with a given object to one of your methods. The most important prerequisite for making this connection is to ensure that your event handler method’s signature matches what the event expects (by implementing the ShapeMouseEventHandler interface). Let’s say you want to invoke a method when the user clicks on a Shape object of some kind. All mouse events, including the click event, requires that the method to be connected have the following four parameters in the given order:
# | Type | Description |
---|---|---|
1. | Shape |
The Shape object that initiated the mouse event |
2. | double |
The x-coordinate at which the event occurred |
3. | double |
The y-coordinate at which the event occurred |
4. | int |
A number identifying the mouse button used |
For example, the following method may be invoked by a mouse event because it implements the necessary four parameter types in the specified order.
public void onPressed(Shape shp, double, x, double y, int button) {
System.out.println("The mouse was pressed at coordinates ("
+ x + "," + y + ")");
}
Every Shape object in DoodlePad inherits a set of methods used to attach mouse events to a suitable method - one method for each mouse event type. Each Shape method takes a single argument: a reference to the method to be invoked when the event occurs. Method references were added to Java in version 8, which is why Java 8 is the minimum required by DoodlePad. Method references are constructed using the
::
binary operator. On the left side of the operator is the name of a class or object that implements a method, and on the right side of the operator is the method to reference.As an example, let’s say your class implements the previous onPress method, and you would like to invoke this method when the mouse is pressed on an Oval Shape object, a reference to which was saved as an instance variable named myOval. The following statement establishes this connection, so that when the mouse is pressed on myOval the onPress method implemented by this will be invoked.
myOval.setMousePressedHandler( this::onPress );
The inherited
setMousePressedHandler(...)
method is what is used to establish the connection for mouse-pressed events.Following is a complete program demonstrating event handing in DoodlePad using method references.
// ToggleButton.java
import doodlepad.*;
// ToggleButton class
public class ToggleButton {
private RoundRect shpButton; // Button Shape
private boolean on; // Button state
// Constructor
public ToggleButton() {
// Create button shape and initialize
shpButton = new RoundRect(100, 100, 75, 50, 20, 20);
on = false; // Starts off
shpButton.setFillColor(200);
// Set button click event handler method
shpButton.setMousePressedHandler(this::onPressed);
}
// Handle mouse-pressed event
private void onPressed(Shape shp, double x, double y, int button) {
toggle();
}
// Toggle button
public void toggle() {
on = !on; // Flip state
if (on) { // Update graphic
shpButton.setFillColor(0, 255, 0);
} else {
shpButton.setFillColor(200);
}
}
public static void main(String[] args) {
// Create a new ToggleButton
ToggleButton myButton = new ToggleButton();
}
}
ToggleButton.java
In this example we implement a simple graphical toggle button class named ToggleButton. For the button graphic we use a RoundRect Shape object. The ToggleButton class tracks its state using an
on
boolean instance variable. Its constructor creates the RoundRect object and initializes its fill color to gray and on = false
implying the button is initially off. The constructor also uses the setMousePressedHandler(…)
method to set the object’s onPressed(…)
method to be invoked when the RoundRect shape is pressed with the mouse. The onPressed(…)
method invokes a toggle()
method that flips the value of on
and then resets the RoundRect fill color based on the current object state.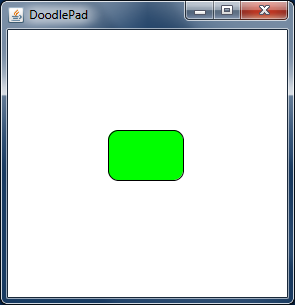
The image on the right shows the output from running this example program and pressing the RoundRect Shape once with the mouse.
Subsection 14.6.2 Shape Mouse Event-Related Methods
A wide variety of mouse events are captured and can be handled in a similar manner. Following is a list of all methods used to connect a mouse event with a Shape. Each method is used to associate a particular mouse event with a custom method. Each of the following methods takes a method reference as its only parameter. Each method reference must identify a method with a signature that matches the previously listed four parameter types in the given order, otherwise the compiler will complain.
Method | Description |
---|---|
public void setMouseClickedHandler(ShapeMouseEventHandler handler) 1 | Set a method to be invoked when the mouse is clicked on a Shape. A mouse-click event occurs when the mouse is pressed and released at the same location. |
public void setMouseDoubleClickedHandler(ShapeMouseEventHandler handler) 2 | Set a method to be invoked when the mouse is double-clicked on a Shape. A mouse-double-clicked event occurs when the mouse is pressed and released at the same location twice in succession. |
public void setMousePressedHandler(ShapeMouseEventHandler handler) 3 | Set a method to be invoked when the mouse is pressed on a Shape. A mouse-pressed event occurs when the mouse button is pressed while over a Shape. |
public void setMouseReleasedHandler(ShapeMouseEventHandler handler) 4 | Set a method to be invoked when the mouse is released on a Shape. A mouse-released event occurs when the mouse button is released while over a Shape. |
public void setMouseMovedHandler(ShapeMouseEventHandler handler) 5 | Set a method to be invoked when a mouse is moved on a Shape. A mouse-moved event occurs when the mouse is moved over a Shape while the mouse button is not pressed. |
public void setMouseDraggedHandler(ShapeMouseEventHandler handler) 6 | Set a method to be invoked when the mouse is dragged on a Shape. A mouse-dragged event occurs when the mouse is moved over a Shape while the mouse button is pressed. |
public void setMouseEnteredHandler(ShapeMouseEventHandler handler) 7 | Set a method to be invoked when the mouse enters a Shape. A mouse-entered event occurs when the mouse is moved from a location outside the bounds of a Shape to a location within the bounds of a Shape. |
public void setMouseExitedHandler(ShapeMouseEventHandler handler) 8 | Set a method to be invoked when the mouse exits a Shape. A mouse-exited event occurs when the mouse is moved from a location within the bounds of a Shape to a location outside the bounds of a Shape. |
Subsection 14.6.3 Other Event-Related Shape Methods
Shapes triggers one additional event beyond the above list of mouse events. The selectionChanged event is triggered whenever the selection status of a Shape changes. For example, if the user clicks on a selectable Shape that is not already selected, the Shape becomes selected, which is indicated by a light gray rectangle drawn around the Shape. If a suitable event handler method is attached to the Shape’s selectionChange event, then as the Shape becomes selected, the event method will be invoked, indicating the Shape object whose selection state changed as well as if the Shape is currently selected. Likewise, if another Shape is clicked causing the currently selected Shape to be unselected, the event handler method will once again be invoked.
Both the method reference and overriding methods of handling triggered selectionChanged events are supported. Event handler methods to be attached to the selectionChanged event trigger using the method reference approach must have a signature with two parameters: Shape and boolean. This implements the ShapeSelectionEventHandler interface. The first parameter indicates the Shape object whose selection changed, and the second parameter indicates if that Shape is currently selected (true) or not (false).
The following two methods are used for handling triggered selectionChanged events. The first method attaches an event handler using the method reference approach. Override the second method to handle the triggered event using the method overriding approach.
Method | Description |
---|---|
public void setSelectionChangedHandler(ShapeSelectionEventHandler handler) 9 | Specify a suitable method to handle selectionChanged events triggered by a Shape object. The method reference must implement the signature defined by the ShapeSelectionEventHandler 10 interface. |
public void onSelectionChanged(boolean selected) 11 | Override this method in a Shape subclass to handle selectionChanged events by overriding the base class method. |
Subsection 14.6.4 Pad Mouse Event-Related Methods
Method | Description |
---|---|
public void setMousePressedHandler(PadMouseEventHandler handler) 12 | Assign an onMousePressed event handler using a method reference. |
public void setMouseReleasedHandler(PadMouseEventHandler handler) 13 | Assign an onMouseReleased event handler using a method reference. |
public void setMouseMovedHandler(PadMouseEventHandler handler) 14 | Assign an onMouseMoved event handler using a method reference. |
public void setMouseClickedHandler(PadMouseEventHandler handler) 15 | Assign an onMouseClicked event handler using a method reference. |
public void setMouseDoubleClickedHandler(PadMouseEventHandler handler) 16 | Assign an onMouseDoubleClicked event handler using a method reference. |
public void setMouseDraggedHandler(PadMouseEventHandler handler) 17 | Assign an onMouseDragged event handler using a method reference. |
public void setMouseEnteredHandler(PadMouseEventHandler handler) 18 | Assign an onMouseEntered event handler using a method reference. |
public void setMouseExitedHandler(PadMouseEventHandler handler) 19 | Assign an onMouseExited event handler using a method reference. |
public void addMouseListener(PadMouseListener o) 20 | Add object to the list of items that are notified on Pad’s mouse events. |
public void removeMouseListener(PadMouseListener o) 21 | Remove object from Pad’s mouse listener list. |
Subsection 14.6.5 Pad Keyboard Event-Related Methods
Method | Description |
---|---|
public void setKeyPressedHandler(Pad.PadKeyEventHandler handler) 22 | Assign an onKeyPressed event handler using a method reference. |
public void setKeyReleasedHandler(Pad.PadKeyEventHandler handler) 23 | Assign an onKeyReleased event handler using a method reference. |
public void setKeyTypedHandler(Pad.PadKeyTypedEventHandler handler) 24 | Assign an onKeyTyped event handler using a method reference. |
public void addKeyListener(PadKeyListener o) 25 | Add object to the list of items that are notified on Pad’s key events. |
public void removeKeyListener(PadKeyListener o) 26 | Remove object from Pad’s key listener list. |
Subsection 14.6.6 Pad Timer Event-Related Methods
Method | Description |
---|---|
public void setTickHandler(Pad.PadTimerEventHandler handler) 27 | Assign an onTick event handler using a method reference. |
public void addTickListener(PadTickListener o) 28 | Add object to the list if items that are notified on Pad’s timer tick action. |
public void removeTickListener(PadTickListener o) 29 | Remove object from Pad’s timer tick action listener list. |
Subsection 14.6.7 Box Driver Example
In the following example a blue Rectangle is created and positioned on a Pad object. The default Pad object’s keyPressed event is handled with a custom method that reacts to the arrow keys being pressed. Only arrow keys are tested; all other keys are ignore. When the down arrow is pressed, the box object’s move(...) method is used to move the box 10 pixels down (by increasing its y-location). Likewise, the remaining three arrow keys move the box left, right or up, depending upon the key pressed. The result is a simple program that can be used to drive a box around the Pad using the arrow keys.
One item to note is the way the key String being pressed is tested. For all arrows keys the keyText String argument passed to the event handler method is compared to both a String describing the key (e.g. "Left") and a symbol (e.g. "←"). An arrow key is identified with a positive match to either the description or symbol String. The reason we test both is that the String passed to the event handler may be different depending upon your operating system. Specifically, Windows sets keyText to key description ("Left") while macOS uses a symbol (e.g. "←"). Give it a try.
// BoxDriver.java
import doodlepad.*;
public class BoxDriver
{
// The box to drive around the Pad
private Rectangle box;
public BoxDriver() {
// Explicitly create a Pad and handle key-pressed event
Pad p = new Pad(600, 600);
p.setKeyPressedHandler( this::onKeyPressed );
// Create ans style the box to drive
box = new Rectangle(100, 100, 50, 50);
box.setFillColor(0, 0, 255);
}
// Key pressed event handler moves box according key pressed
public void onKeyPressed(Pad p, String keyText, String keyMods) {
if (keyText.equals("Left") || keyText.equals("←")) {
box.move(-10, 0);
} else if (keyText.equals("Right") || keyText.equals("→")) {
box.move(10, 0);
} else if (keyText.equals("Up") || keyText.equals("↑")) {
box.move(0, -10);
} else if (keyText.equals("Down") || keyText.equals("↓")) {
box.move(0, 10);
}
}
public static void main(String[] args) {
BoxDriver myBoxDriver = new BoxDriver();
}
}
BoxDriver.java
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseClickedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseDoubleClickedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMousePressedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseReleasedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseMovedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseDraggedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseEnteredHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setMouseExitedHandler-doodlepad.Shape.ShapeMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.html#setSelectionChangedHandler-doodlepad.Shape.ShapeSelectionEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Shape.ShapeSelectionEventHandler.html
doodlepad.org/dist/javadoc/doodlepad/Shape.html#onSelectionChanged-boolean-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMousePressedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseReleasedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseMovedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseClickedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseDoubleClickedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseDraggedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseEnteredHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setMouseExitedHandler-doodlepad.Pad.PadMouseEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#addMouseListener-doodlepad.PadMouseListener-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#removeMouseListener-doodlepad.PadMouseListener-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setKeyPressedHandler-doodlepad.Pad.PadKeyEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setKeyReleasedHandler-doodlepad.Pad.PadKeyEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setKeyTypedHandler-doodlepad.Pad.PadKeyTypedEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#addKeyListener-doodlepad.PadKeyListener-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#removeKeyListener-doodlepad.PadKeyListener-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#setTickHandler-doodlepad.Pad.PadTimerEventHandler-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#addTickListener-doodlepad.PadTickListener-
doodlepad.org/dist/javadoc/doodlepad/Pad.html#removeTickListener-doodlepad.PadTickListener-