Section 8.6 Multi-File Programs
Because Java limits a single Java file to one public class definition, it follows that the source code of a program involving two or more custom public classes must be made up of multiple Java source code files. Recall that there is no limit to the number of classes defined within a single source code file. But, only one of those classes may be
public
and its name must match the file name. Any number of helper classes may be defined in single file, which are used internally by the public class in the file.Subsection 8.6.1 Compiling Multi-file Programs
A program involving multiple source code files is compiled by simply listing all source files after the
javac
command. The Java compiler identifies where to find each class by the name of the file. For example, if we have defined two custom classes in our program, Class1.java
and Class2.java
, then all must be compiled together using a command like the following.javac Class1.java Class2.java
Simply list all source code file names after the
javac
command. If additional classes are defined in a JAR file, like doodlepad.jar
, then add the standard classpath option (-cp doodlepad.jar
) to the command.javac -cp doodlepad.jar Class1.java Class2.java
The compiler searches and finds all classes that it needs to produce compiled class files. The compiler will complain if it cannot find a class. Note that you will see a compiled
.class
file produced by the compiler for every class that you define, even if those classes are not public.Subsection 8.6.2 The Driver Class
In which class file should the overall
main(…)
method be placed — the one that initializes all classes and starts running your program? It may not always be obvious. After all, for our individual class definitions to be reusable, it does not make a lot of sense to use a class’s main(…)
method to start a larger application. Furthermore, in Section 8.3 we reserved the main(…)
method to run tests on our custom class.One approach is to author a separate class that defines only this top-level
main(…)
method which sets up and starts the larger program. In a sense, this main(…)
method drives the rest of your program, hence the term Driver Class. In fact, you may define several Driver Classes in a program, each with a different purpose, and invoke each separately by specifying the name of the Driver Class of interest when running your program using the java
launcher command.Let’s say that we want to build a grid of multiple LED objects as a virtual panel of indicator lights. The panel consists of two rows of four lights, one of each color in each row. We can use our previous LED class and the following Driver class to build our virtual panel.
The LEDPanel class in Listing 8.6.1 implements only the
main(…)
method which sets up and runs the program. First in the main(…)
method a Pad object is created directly with a title and custom dimensions. Rather than declare eight LED variables, an array of LED objects named leds
is declared. Each element of the leds
array is assigned to a new LED object with a certain color. Note that we are using an LED class with the updated constructor overload that takes an additional color parameter used to initialize the LED with color.// LEDPanel.java
import doodlepad.*;
// Driver class
public class LEDPanel {
public static void main(String[] args) {
// Create a Pad object with title and custom dimensions
Pad panel = new Pad("LED Panel", 550, 350);
// Store LED objects in a 2D array matching layout
LED[][] leds = new LED[2][4];
// Create and position all LED objects on panel
leds[0][0] = new LED(100, 100, LEDColor.RED);
leds[0][1] = new LED(200, 100, LEDColor.GREEN);
leds[0][2] = new LED(300, 100, LEDColor.BLUE);
leds[0][3] = new LED(400, 100, LEDColor.YELLOW);
leds[1][0] = new LED(100, 200, LEDColor.RED);
leds[1][1] = new LED(200, 200, LEDColor.GREEN);
leds[1][2] = new LED(300, 200, LEDColor.BLUE);
leds[1][3] = new LED(400, 200, LEDColor.YELLOW);
// Turn on all LED objects
for (int r=0; r<leds.length; r++) {
for (int c=0; c<leds[r].length; c++) {
leds[r][c].turnOn();
}
}
}
}
LEDPanel.java
When compiling, all classes are specified in a sequence following the
javac
command. Only the Driver Class is named when running the program with java
because the driver class contains the main(…)
method which starts the program.// All Java files are compiled together javac -cp .;doodlepad.jar LEDPanel.java LED.java // Only the Driver Class name is used when run java -cp .;doodlepad.jar LEDPanel
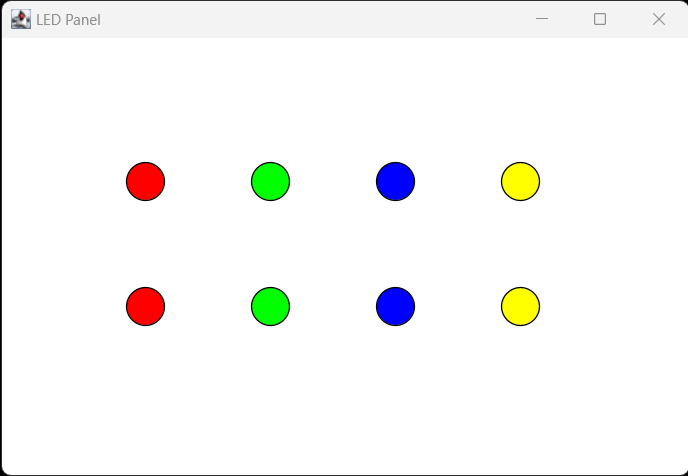
LEDPanel.java